Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial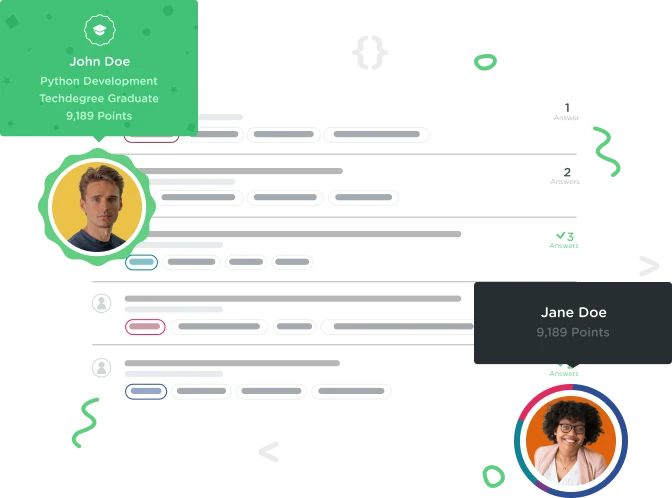
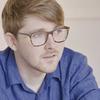
Alex Ryan
1,211 PointsInitialize the field 'numSides' to 4
I got most of the way through this challenge but got stuck on the part where I need to initialize numSides to 4 in the base constructor. After trying everything I could think of and searching forums I still couldn't find the solution.
The error message I keep getting after checking the code is: "Did you initialize the field 'numSides' to 4 in the base constructor?"
Can anyone spot where I'm going wrong?
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(int numSides)
{
SideLength = sideLength;
NumSides = 4;
}
}
}
2 Answers
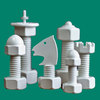
Steven Parker
230,274 PointsSince the base ("Polygon") constructor uses its argument as the value for the parameter "numSides", anything you pass to the base will be used to initialize the field NumSides.
So to "Use base to initialize NumSides to 4" you would call "base(4)
".
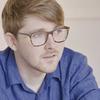
Alex Ryan
1,211 PointsAh I see, thank you so much, it's working now!
Alex Ryan
1,211 PointsAlex Ryan
1,211 PointsI replaced "NumSides = 4" with "base(4)", which is what I think you were saying to do but I still get the same error message.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsYou do need to remove the reference to "NumSides" from "Square"s constructor, but what I was suggesting about "base" is this replacement: