Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial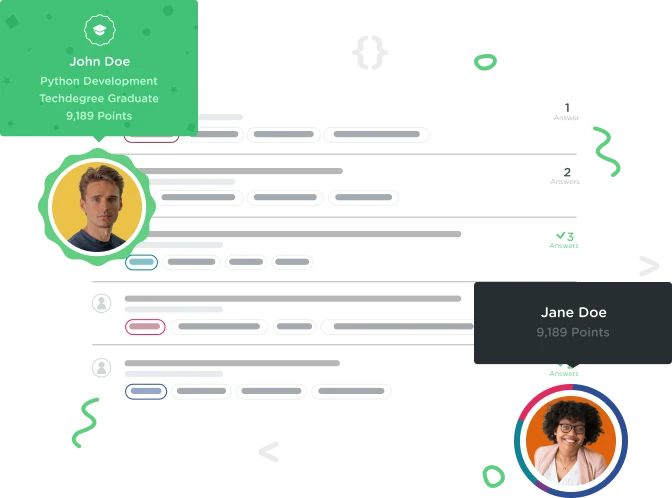
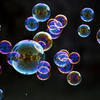
Jialong Zhang
9,821 PointsInitializer Delegation Unknown second initializer error
struct Point{
let x: Int = 0
let y: Int = 0
}
struct Size{
let width: Int = 0
let height: Int = 0
}
struct Rectangle{
var origin = Point()
var size = Size()
init(origin: Point, size: Size){
self.origin = origin
self.size = size
}
init(x: Int, y: Int, width: Int, height: Int){
let origin = Point(x: x, y: y)
let size = Size(width: width, height: height)
self.init(origin: origin, size: size)
}
}
3 Answers
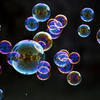
Jialong Zhang
9,821 Pointsthank you for your answer. I just wondering about the wrong codes. I followed the exact same code in the video.

Heidi Puk Hermann
33,366 Pointsso, I just redid the video, and it is "my point 1" thats different between your code and his. He uses variables instead of constants. In that case, the rest of your code should work fine then. :)
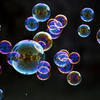
Jialong Zhang
9,821 Pointsthanks
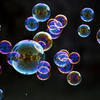
Jialong Zhang
9,821 PointsThanks for your answer. How can I mark your answer as best answer
Heidi Puk Hermann
33,366 PointsHeidi Puk Hermann
33,366 Pointsso, there is a few error in your code.
I have attached a working code, where the errors have been fixed :)