Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial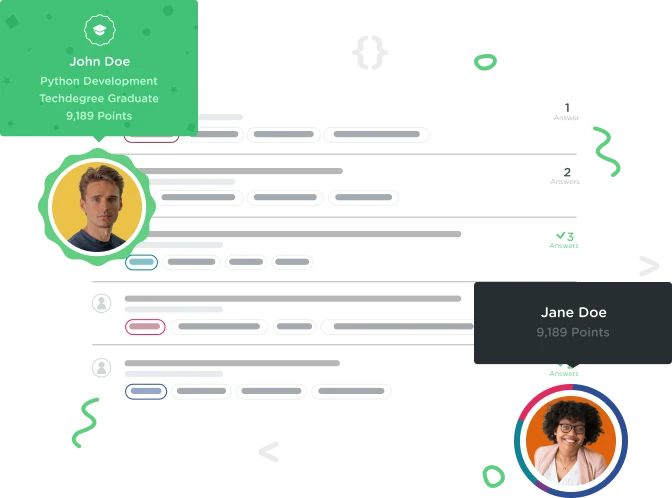

Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsInitializing a set in the constructor.
I'm struggling so far. I've tried many different random attempts to initialize mTags as a set in the constructor. This was the last attempt I did, just randomly adding strings to see if I can get it to initialize. Need some help.
package com.example.model;
import java.util.List;
import java.util.Set;
import java.util.HashSet;
public class Course {
private String mTitle;
private Set<String> mTags;
public Course(String title) {
mTitle = title;
// TODO: initialize the set mTags
Set<String> mTags = new HashSet<String>();
mTags.add("a b");
mTags.add("b a");
}
public void addTag(String tag) {
// TODO: add the tag
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
}
public boolean hasTag(String tag) {
// TODO: Return whether or not the tag has been added
return false;
}
public String getTitle() {
return mTitle;
}
}
2 Answers
Christopher Augg
21,223 PointsKyle,
Great job at figuring it out.
We should not use Set mTags = new HashSet(); in the constructor in this particular case because we have a declaration for a mTags member variable already:
private Set<String> mTags;
Using Set mTags = new HashSet(); in the constructor would make it local to the constructor. Therefore, the mTags member variable outside the constructor would never be initialized. Of course, we could declare and initialize our private member variable outside the constructor. However, the challenge asked for it to be initialized within the constructor.
private Set<String> mTags = new HashSet<String>();
Lastly, we can declare a Set without a type as you did.
private Set mTags = new HashSet();
However, you will get a warning that Set is a rawType. While it would compile with the warning, it can lead to exceptions being thrown. Please refer to rawType Oracle Documentation.
I hope that clears it it up for you. Please let me know if you have any other questions.
Regards,
Chris

Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsNevermind, I found it. Incase anyone was wondering for the question. It was the challenge 1 of 3 in the Java Data Structures. How you initialize a set variable is like this:
mTags = new HashSet<String>();
That's it. What I don't understand too well is why Set<String> mTags = new HashSet<String>(); doesn't work? Anyone know that answer?

Sid b
15,346 Pointsi think becaue it's declared in the class already