Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial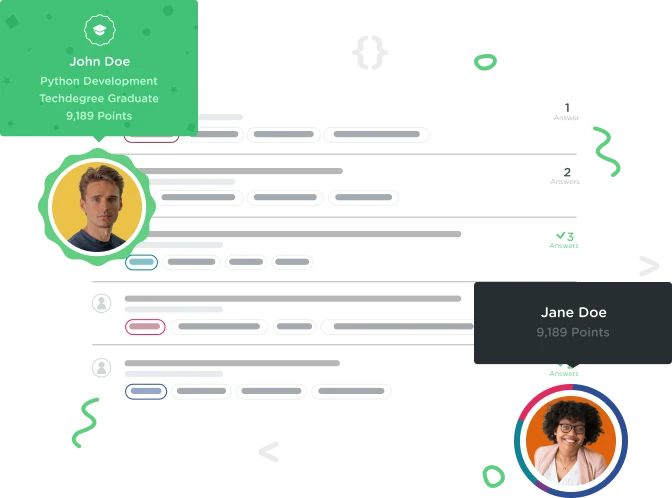
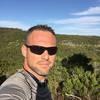
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsInitializing the value of a key
Okay this is the last of my own practice apps I am trying to finish before I start the tech degree program. Here I am trying to search through a table view. This has me stumped. I just can't seem to figure out how to initialize the value of "name" so that I can search it.
var results = WOD(dictionary:["name": ""])
Just doesn't seem to do it. (https://github.com/Brandon-316/WOD-Guide)
Library sample code:
import Foundation
struct BenchmarkWODs {
static let library = [
[
"name": "Angie",
"exercises": "100 Pull-ups/n100 Push-ups/n100 Sit-ups/n100 Squats",
"description": "For Time Complete all reps of each exercise before moving to the next."
],
[
"name": "Barbara",
"exercises": "20 Pull-ups\n30 Push-ups\n40 Sit-ups50 Squats\n\nRest precisely three minutes between each round.",
"description": "5 rounds, time each round"
],
[
"name": "Chelsea",
"exercises": "5 Pull-ups\n10 Push-ups\n15 Squats",
"description": "Each min on the min for 30 min"
],
[
"name": "Cindy",
"exercises": "5 Pull-ups\n10 Push-ups\n15 Squats",
"description": "As many rounds as possible in 20 min"
]
]
}
Detail file code:
import Foundation
import UIKit
struct WOD {
var name: String?
var exercise: String?
var description: String?
init(dictionary: [String: String]) {
name = dictionary["name"]
exercise = dictionary["exercises"]
description = dictionary["description"]
}
}
ViewController code:
import UIKit
class BenchmarkWODViewController: UITableViewController,
UISearchResultsUpdating {
var wodList = [WOD]()
var searchController : UISearchController?
var resultsController = UITableViewController()
var filteredWODS = [String]()
var results = WOD(dictionary:["name": ""])
override func viewDidLoad() {
super.viewDidLoad()
for wodData in BenchmarkWODs.library {
let wod = WOD(dictionary: wodData)
wodList.append(wod)
}
// Search Bar
self.searchController = UISearchController(searchResultsController: self.resultsController)
self.tableView.tableHeaderView = self.searchController?.searchBar
self.searchController?.searchResultsUpdater = self
}
// Search Bar Function
func updateSearchResultsForSearchController(searchController: UISearchController) {
let result = results.name
filteredWODS.append(result!)
filteredWODS = self.filteredWODS.filter { (result:String) -> Bool in
return true
}
self.resultsController.tableView.reloadData()
}
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 1
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return wodList.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
let cell = tableView.dequeueReusableCellWithIdentifier("BenchmarkCell", forIndexPath: indexPath) as UITableViewCell
let wod = wodList[indexPath.row]
if let wodName = wod.name {
cell.textLabel?.text = wodName
}
return cell
}
}