Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial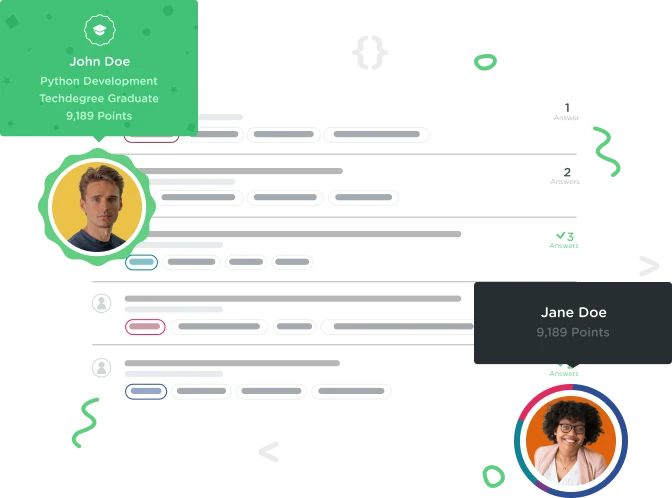
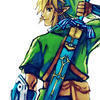
Dhruv Patel
8,287 Points@InjectView in HourAdapter
Is it possible for me to use @InjectView in my HourAdapter.HourViewHolder and if it is then where would I go about to add the ButterKnife.inject(this) call
3 Answers
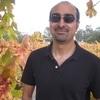
Kourosh Raeen
23,733 PointsYes, it is. Call ButterKnife.inject(this, itemView) inside the HourViewHolder constructor, where all the findViewById calls are. Here's the code:
package com.teamtreehouse.stormy.adapters;
import android.content.Context;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.teamtreehouse.stormy.R;
import com.teamtreehouse.stormy.weather.Hour;
import butterknife.ButterKnife;
import butterknife.InjectView;
/**
* Created by Kourosh on 7/21/2015.
*/
public class HourAdapter extends RecyclerView.Adapter<HourAdapter.HourViewHolder> {
private Hour[] mHours;
private Context mContext;
public HourAdapter(Context context, Hour[] hours) {
mContext = context;
mHours = hours;
}
@Override
public HourViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.hourly_list_item, parent, false);
HourViewHolder hourViewHolder = new HourViewHolder(view);
return hourViewHolder;
}
@Override
public void onBindViewHolder(HourViewHolder holder, int position) {
holder.bindHour(mHours[position]);
}
@Override
public int getItemCount() {
return mHours.length;
}
public class HourViewHolder extends RecyclerView.ViewHolder
implements View.OnClickListener{
//public TextView mTimeLabel;
//public TextView mSummaryLabel;
//public TextView mTemperatureLabel;
//public ImageView mIconImageView;
@InjectView(R.id.timeLabel)
TextView mTimeLabel;
@InjectView(R.id.summaryLabel)
TextView mSummaryLabel;
@InjectView(R.id.temperatureLabel)
TextView mTemperatureLabel;
@InjectView(R.id.iconImageView)
ImageView mIconImageView;
public HourViewHolder(View itemView) {
super(itemView);
//mTimeLabel = (TextView) itemView.findViewById(R.id.timeLabel);
//mSummaryLabel = (TextView) itemView.findViewById(R.id.summaryLabel);
//mTemperatureLabel = (TextView) itemView.findViewById(R.id.temperatureLabel);
//mIconImageView = (ImageView) itemView.findViewById(R.id.iconImageView);
ButterKnife.inject(this, itemView);
itemView.setOnClickListener(this);
}
public void bindHour(Hour hour) {
mTimeLabel.setText(hour.getHour());
mSummaryLabel.setText(hour.getSummary());
mTemperatureLabel.setText(hour.getTemperature() + "");
mIconImageView.setImageResource(hour.getIconId());
}
@Override
public void onClick(View v) {
String time = mTimeLabel.getText().toString();
String temperature = mTemperatureLabel.getText().toString();
String summary = mSummaryLabel.getText().toString();
String message = String.format("At %s it will be %s and %s",
time,
temperature,
summary);
Toast.makeText(mContext, message, Toast.LENGTH_LONG).show();
}
}
}
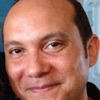
Peter Corletto
2,682 PointsIt is not @InjectView anymore. It is now @Bind. Also, use ButterKnife.bind(this)
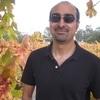
Kourosh Raeen
23,733 PointsHi Peter - @Bind is for a newer version of the Butterknife library. The one used for this course is an older version that uses @InjectView.
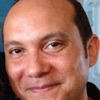
Peter Corletto
2,682 PointsOh good to know. Thank you.