Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial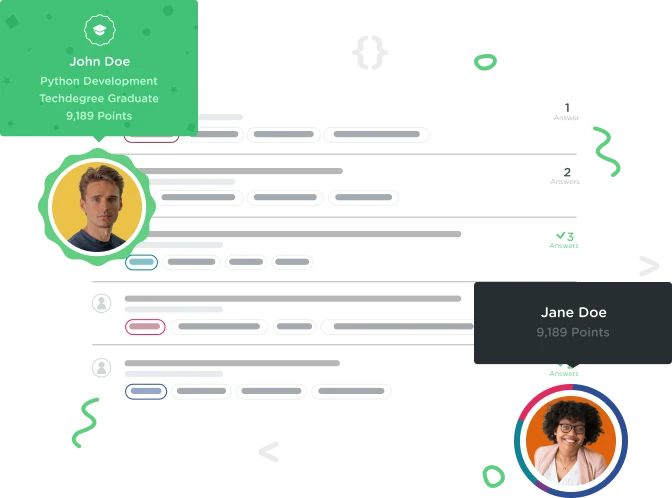

Allon de Veen
2,284 Pointsinner class
What's the deal with that question about inner class? The course hasn't covered that yet. I can't see what I did wrong.
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides = 4;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(numSides)
{
}
}
}
2 Answers
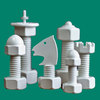
Steven Parker
231,268 PointsI don't think the challenge mentions "inner class". But it does say that the new field "SideLength" should be initialized using the constructor. That means it should have a value set similar to how "Polygon" sets "NumSides" in its constructor. So you still need to do that part.
Also, when they say "Use base to initialize NumSides to 4" they mean you should literally pass a 4 to base. It will handle setting "NumSides" for you.

Allon de Veen
2,284 PointsThis is the assignment:
The Polygon class represents a 2 dimensional shape. It has a public field named NumSides. Create a new type of polygon called Square. It should have a public readonly field named SideLength thatβs initialized using the constructor. Use base to initialize NumSides to 4.
This is my code:
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = 4;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(numSides)
{
SideLength = 4;
}
}
}
This is the error message I am getting:
Bummer! Did you define your Square class as an inner class of the Polygon class? Be sure to define the Square class before or after the definition for the Polygon class.
As you can see the challenge didn't mention inner class but the error message did. With this new code it still doesn't work.
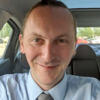
Andrew Sykes
9,286 Pointsnamespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
}
The NumSides parameter is satisfied during constructing this new sub-class of Square by passing '4' directly to base. The new parameter "SideLength" is unique to your subclass Square.