Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial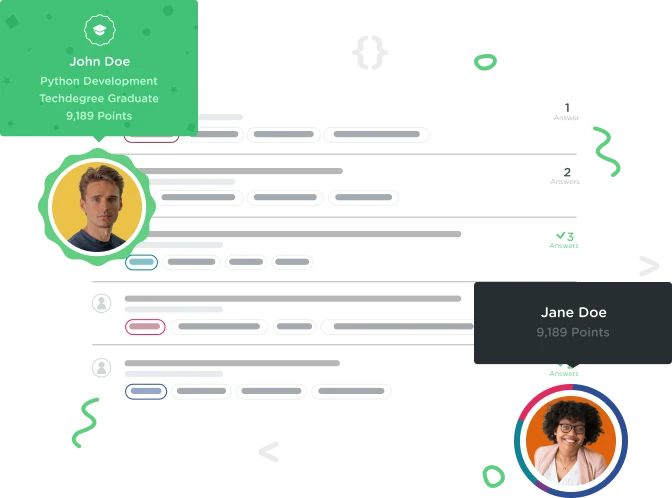

Jeremy Justus
2,910 PointsIn-place replacement while iterating
So, while my code essentially works, I've been having trouble with the final step. The issue seems to be with my for loop. I'm trying to do an in-place replacement of the current value, and it isn't working the way I had hoped.
def vowel_strip(s):
s_list = list(s)
vowels = 'aeiou'
for l in s_list:
if l in vowels:
s_list.remove(l)
s_list = ''.join(s_list)
return s_list
print("\nPlease enter a list of words to be stripped of all its precious vowels:")
s = input("> ")
s = s.lower()
s = s.split()
for w in s:
w = vowel_strip(w)
w = w.capitalize()
print(w)
print("Here you go: {} ".format(s))
The print(w) within the loop shows me that it's being stripped and capitalized like I want, but the assignment back to the list member doesn't seem to happen. I know I could simply change it so that it works essentially like Kenneth's explanation, but for future reference is there an easy way to do what the above code is attempting? Thanks.
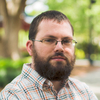
Kenneth Love
Treehouse Guest Teacherraw_input()
is only in Python 2. We use Python 3 here at Treehouse, so we always use input()
.
2 Answers
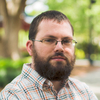
Kenneth Love
Treehouse Guest TeacherIt's generally not considered a great idea to modify a list while you're looping through it. I'd suggest having a second, blank list that you append your vowel-stripped items into and then returning that list in the end.

Jeremy Justus
2,910 PointsThanks for all the replies!
beven nyamande
9,575 Pointsbeven nyamande
9,575 Pointsi would suggest using raw_input than input() , heard there are different since input() is mainly for capturing numbers while raw_input is for strings,i dont know if this helps but will try doing your program.I have also been adviced to use variable names easy to debug consider my code below