Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial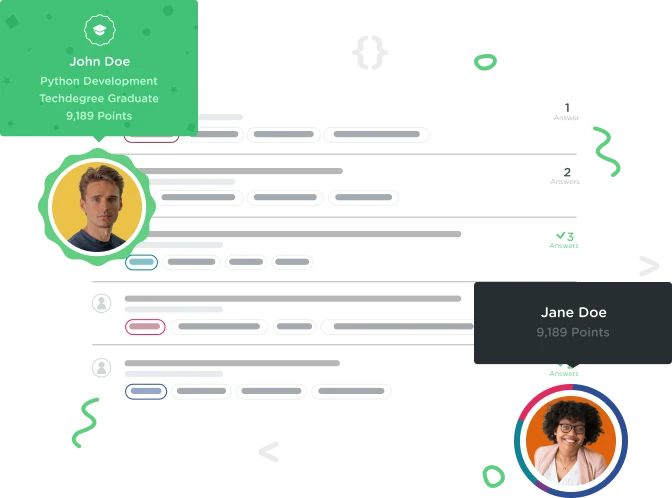
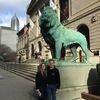
Matthew Beiswenger
6,814 PointsInput is returning a zero
I'm trying to do a little practicing on my own, combining elements of Jon Duckett's JavaScript and JQuery book with those of Treehouse's JavaScript Basics track. Basically the program allows someone to type in text and see how much it would cost to make a sign with that text.
When I try to copy the text from the input element and place it next to custom sign, my input.value returns a zero. Any help would be greatly appreciated with figuring this out
HTML:
<html>
<head>
<title>Duckett Chapter 2 Example</title>
<link href="styles.css" rel="stylesheet "type="text/css" >
</head>
<body>
<h2 id="greeting">Howdy Molly, please check your order: </h2>
<div>
<ul id="left-col">
<li><span>Custom Sign: </span> </li>
<li><span>Total Tiles: </span></li>
<li><span>Subtotal: </span></li>
<li><span>Shipping: </span></li>
<li><span>Grand Total: </span></li>
</ul>
<ul id="right-col">
<li id="customSign">Montague Hotel</li>
<li id="totalTiles">14</li>
<li id="subtotal">$70</li>
<li id="shipping">$7</li>
<li id="grandTotal">$77</li>
</ul>
<div id="input-form">
<input id="textInput" type="text">
<button id="myButton">Calculate</button>
</div>
</div>
<script src="scripts.js"></script>
</body>
</html>
JAVASCRIPT:
var btnCalc = document.getElementById('myButton');
// Here's where I have the issue
var textInput = document.getElementById('textInput').value;
var customSign = document.getElementById('customSign');
var totalTiles = textInput.length;
var subtotal = totalTiles * 5;
var shipping = 7;
var grandTotal = subtotal + shipping;
btnCalc.addEventListener('click', Calculate);
function Calculate() {
// and here
document.getElementById('customSign').innerHTML = textInput;
document.getElementById('totalTiles').textContent = totalTiles;
document.getElementById('subtotal').textContent = subtotal;
document.getElementById('shipping').textContent = shipping;
document.getElementById('grandTotal').textContent = grandTotal;
}
CSS (although I don't think it's relevant):
h2 {
text-align: center;
}
span {
font-weight: bold;
}
div {
margin: 0 auto;
width: 400px;
overflow: auto;
}
ul {
list-style-type: none;
}
#right-col {
float: right;
}
#left-col {
float:left;
}
#input-form {
text-align: center;
}
1 Answer
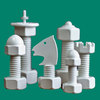
Steven Parker
230,688 PointsYou don't get the current input box value.
When the page is first loaded, you assign the variable textInput to what's currently in the box. At that point, it's empty. Then when the button is clicked you transfer that empty string to the customSign element.
For what you're trying to do, I think you'll want to sample the input fresh when the button is clicked. And also remember to convert the string into a number if you plan to do any calculations on it. And of course, the calculations would also need to be done inside the click handler.