Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial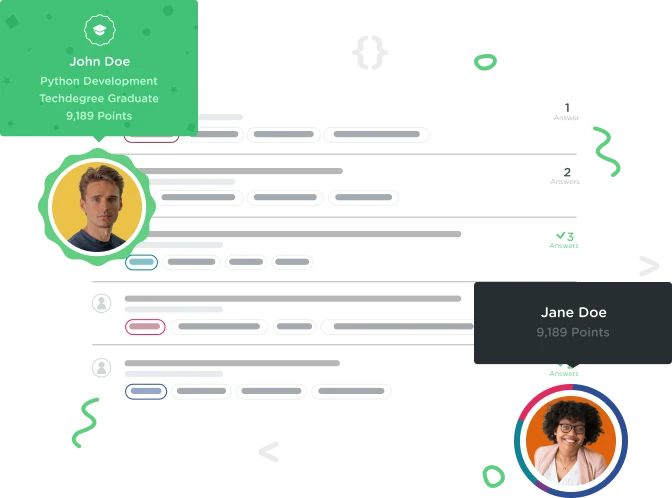
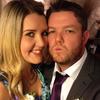
Matthew Thompson
4,500 PointsInput print extra credit
Hi, Im just working my way through the Ruby on rails track and completed the extra credit for getting an input name and outputting the length. It works but I was wondering if anyone could explain how the class initialize works.
It isn't really made clear why we do the initialize part and then why we call it after the functions.
#name
#number of characters
#if length is over 25 print out a message
class NameLength
def initialize()
end
def name
print "What is your name? "
name = gets.chomp
@lengthName = name.length
if @lengthName > 25
puts "Your name has too many characters.. Sorry Dude!"
else
puts "Hi #{name}, you have #{@lengthName} characters in your name."
end
end
end
your_name = NameLength.new()
your_name.class # => Namelength
your_name.name ```
2 Answers

Tim Knight
28,888 PointsMatthew,
I just thought I'd try your solution a little differently. This takes advantage of using the initialize method and separates out the logic of checking the length of the name to a different method. I also pulled out the question of the name because it seems a little weird for me for that to be in the length class, but that's just my opinion. One of the things I did a lot when I first started Ruby was create variables were I didn't really need them. It could be said too that I don't really need to create the instance variable in the initialize method here, but it's for learning purposes.
Another thing that's common in Ruby is that where you might use camel case in JavaScript, Rubyists tend to do snake case—basically, just use underscores. So @lengthName would be @length_name. Just offering a little feedback.
class NameLength
def initialize(name)
@name = name
check_length(@name)
end
def check_length(name)
length = name.length
if length > 25
puts "Your name has too many characters.. Sorry Dude!"
else
puts "Hi #{name}, you have #{length} characters in your name."
end
end
end
puts "What is your name?"
NameLength.new(gets.chomp)

ARNOLD SANDERS
5,266 PointsThe initialize method is a common function used throughout all OO languages. Basically you use it to assign initial values to the OBJECTS that you INSTANTIATE from outside of the class. You INSTANTIATE the new objects by calling object = Class.new( value ) This will pass your value off to the initialize method where it will be assigned to instance variables that you created within it. These values that you assign to your new object will now be available for use within all the instance methods of the class object.
In laymans: My first name is Arnold, I am an object of the Human Class. At birth I was Instantiated with the name Arnold. Now my first name is available for use as apart of the human class. lol
Matthew Thompson
4,500 PointsMatthew Thompson
4,500 PointsThanks, this makes a lot more sense to me. I like how you use the input and pass it as a parameter into the the initialize method. (hope I used the terms correctly here)
Thanks for the advice.