Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial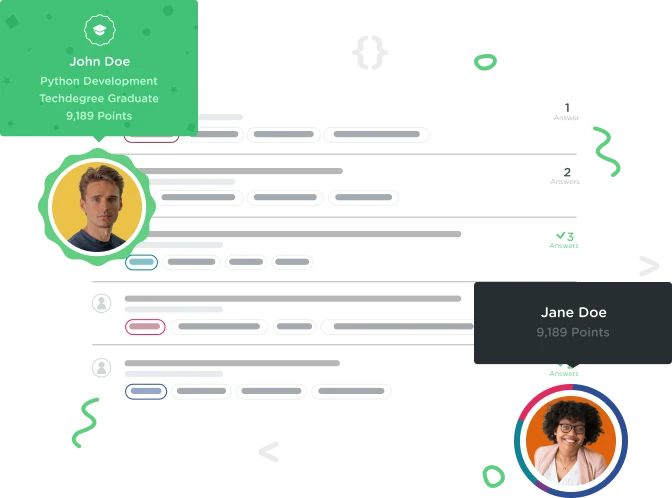
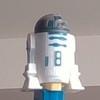
Teacher Russell
16,873 PointsInserting a node
Am I crazy or is that an error on line 80 in the inserting a node video? It should be current.next_node, right?
2 Answers
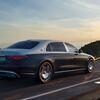
Balazs Peak
46,160 PointsYou are right. Nice move. Attention to details is a superpower when it comes to programming. :D
(Note: probably the video has been left accidentally with the error in it. If you look at the provided source code for the solution in the notes 2 steps after that video, you can see that it's already fixed there. Reference: https://teamtreehouse.com/library/introduction-to-data-structures/building-a-linked-list/linked-lists-operations )
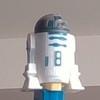
Teacher Russell
16,873 PointsI've seen a couple of inconsistencies between video and notes, yes. I'm going to say it's a testament to Pasan's teaching ability, that even I can spot errors in someone else's code, in a language I don't know, when I'm not only not looking for them, but assuming what I'm looking at is correct. Go Pasan:) Of course, it COULD be my developing super powers, but I think I just got lucky this time. Hopefully someone can add a note in the teacher's notes? It's already a little tricky learning this stuff in a strange language. It might throw off other newbies. Or maybe they'll just see your little chat here. Thanks for the quick reply earlier.
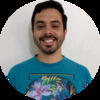
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsI'm not sure, but I think there's another error in the insert method of the Linked List.
Let's suppose we don't have any values in the linked list. This means that the __count property would be 0. If we want to add a node at index zero, we should be able to, shouldn't we? But we won't because of the first if statement which will throw an error:
def insert(self, data, index):
"""
Inserts a new Node containing data at index position
Insertion takes O(1) time but finding node at insertion point takes
O(n) time.
Takes overall O(n) time.
"""
if index >= self.__count: # An error will be thrown
raise IndexError('index out of range')
if index == 0: # This should run, but it won't because an error was thrown
self.add(data)
return
if index > 0:
new = Node(data)
position = index
current = self.head
while position > 1:
current = current.next_node
position -= 1
prev_node = current
next_node = current.next_node
prev_node.next_node = new
new.next_node = next_node
self.__count += 1
I think if the second if statement was moved to be the first one, the algorithm would be correct.

Aaron Uusitalo
4,405 PointsGood catch. That jumped out at me, too. I didn't recall any variable being declared called 'node' (as opposed to Node class), so that's a red flag.

Harald N
15,843 PointsThanks, I'm taking this course, but my main language is Javascript not Python, so wasn't sure if I did something wrong or if the code was wrong.
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsNice catch Teacher Russell
Tagging: Pasan Premaratne