Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial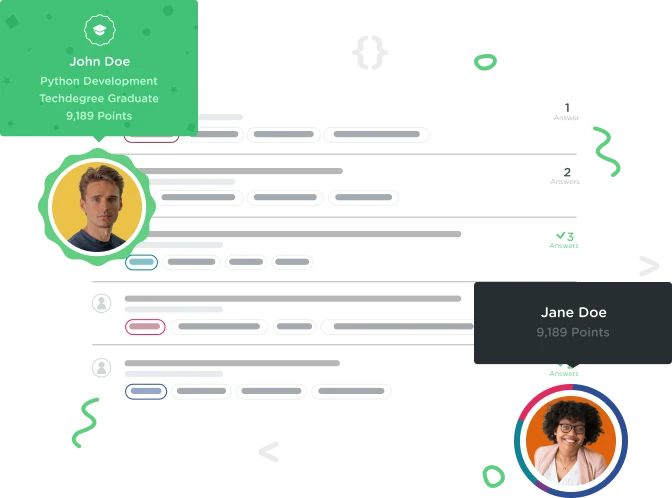

Abson Chifodya
11,817 PointsInside the Student class, create an empty setter method called "major()"
Inside the Student class, create an empty setter method called "major()"
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
set major() {
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
}
var student = new Student(3.9, 60);
3 Answers

Martin Lecke
14,385 PointsA set
is used to change a value therefore it also must recieve a new value of some kind. So you need one parameter for your method.
set major(name) {
}
If theres no parameter it should be a get
method since it should return a value for you.
(Well actually you can change values with both Set and a Get but on a getter you dont recieve your change from a parameter).

Starky Paulino
Front End Web Development Techdegree Student 6,398 Pointsclass Student { constructor(gpa, credits){ this.gpa = gpa; this.credits = credits; }
stringGPA() {
return this.gpa.toString();
}
set major(name) {
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
}
var student = new Student(3.9, 60);

Joe Beltramo
Courses Plus Student 22,191 PointsRead the error provided by the editor
Bummer! Setter must have exactly one formal parameter.
This means that you must provide a parameter for the major
setter