Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial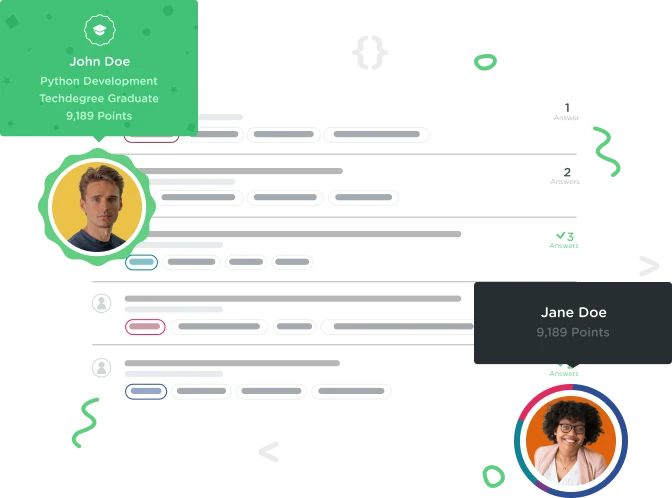

Farhan Hussain
13,928 Points'Instance' Clarification - someone help!
Hi I'm learning iOS and going through the courses and currently on classes. A word that keeps coming back again and again is 'instance'. Such as 'instance of a class' or 'instance of struct'. I have watched the videos and I feel like I know some of it but then I don't really know it - I'm confused.
Can someone please explain to me what does 'instance' actually mean? Like what does it exactly do?
Any answer would be greatly appreciated.
2 Answers
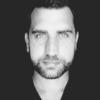
Jhoan Arango
14,575 PointsHello:
An instance is basically an object in its full implementation. A class is a blue print of the object you want to create, and the instance of this class is the "object" in its final stage. Once that instance is created, you can consider it an object, which you can manipulate later by changing its properties.
For example:
// The blueprint of a car object.
class Car {
// The properties
var model: String
var color: Int
var wheels: Int
// The initializer to be able to create the instance ( object )
init(model: String, color: String, wheels: Int){
self.model = model
self.color = color
self.wheels = wheels
}
}
// Creating an instance of Car. ( Basically we create an object )
var fordFiesta = Car(model: "Fiesta", color: "Blue", wheels: 4)
fordFiesta // is the instance
// Just like any other "object" you can change it's properties
fordFiesta.color = "Black"
I hope this gives you an idea of what an instance is.
Good luck

Zachary Kaufman
1,463 PointsI am still new to iOS so I could be wrong, but I think an instance is just an example of when something is used. So an instance of a variable would be
var name = Zach
and
var name = Christian
are both instances of variables. Instances of classes and structs are just times when they are used. Like I said, I could be wrong but I think thats what it means. I hope this helps!
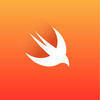
Steven Deutsch
21,046 PointsLike Jhoan said above, classes and structures are the models of what an instance will look like. The actual instance is what is created from that model. Variables and constants are containers which we store instances in. If an instance is stored in a variable, it is mutable. If an instance is stored in a constant, it is immutable. This way we can refer to those containers throughout our code and use their values.
Farhan Hussain
13,928 PointsFarhan Hussain
13,928 PointsThank you so much this helped me a lot in understanding it.
Let me just repeat this to you so I'm sure what I understand is correct. So basically instance is an object that we create using structs and classes?
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsYes..