Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial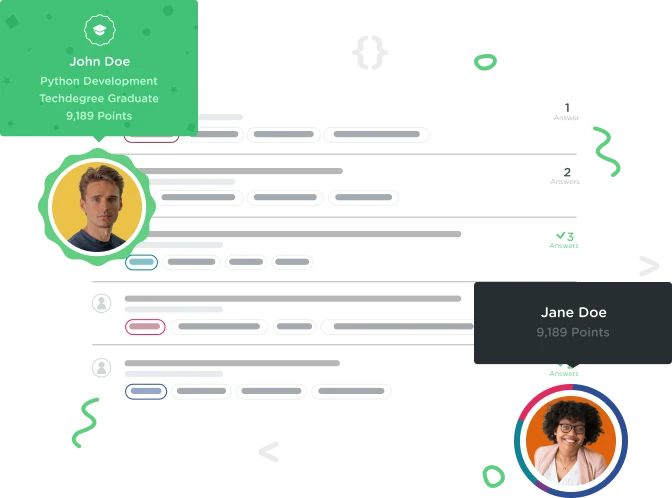

Matt Conway
1,572 PointsInstance for structure
Got the type String, but cannot put a variable under "tag". It doesn't say in instructions what type it is. Nothing works!
Challenge Task 1 of 2
In the editor you've been provided with a Tag type. Create a struct named Post with three stored properties: title of type String author of type String tag of type Tag
Create an instance of Post and assign it to a constant
named/Users/mattconway/Desktop/Screen Shot 2016-01-28 at 5.40.31 PM.png firstPost.
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
}
let firstPost = Post(title: "Treehouse", author: "Pasan", tag: x)
5 Answers
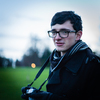
Matthew Spear
14,534 PointsYou need to construct an instance of the tag in the same way in which you construct the Post:
let tagX = Tag(name: "Tag X")
That variable can then be passed into the Post instance since the constant tagX is of type Tag.
Hope that helps, Matt

Matthew Delange
26,584 PointsHey Matt Conway, Not sure if this has been answered correctly for you yet but this worked for me............
struct Tag { let name: String } struct Post { let title: String let author: String let tag: Tag func description() -> String { return "(title) by (author). Filed under (tag.name)" } } let firstPost = Post(title: "iOSDevelopment", author: "Apple", tag: Tag(name: "Swift")) let postDescription = firstPost.description()

alex banaga
310 PointsThank you for this!
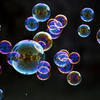
Jialong Zhang
9,821 Pointsstruct Tag {
let name: String
}
struct Post{
let title: String
let author: String
let tag: Tag
}
let firstPost = Post(title: "Bird", author: "May", tag: Tag(name: "tag"))

Lydia Chandrahirawati
6,336 Pointsstruct Tag {
let name: String
}
struct Post {
var title: String
var author: String
var tag: Tag
func description() -> String {
return("\(title) by \(author). Filed under \(tag.name)")
}
}
let firstPost = Post(title: "iOS Development", author: "Apple", tag: Tag(name: "swift"))
let postDescription = firstPost.description()
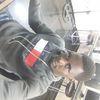
Kellington Chidza
866 Pointsstruct Tag { let name: String }
struct Post { var title: String var author: String var tag: Tag
func description() -> String {
return("\(title) by \(author). Filed under \(tag.name)")
}
}
let firstPost = Post(title: "iOS Development", author: "Apple", tag: Tag(name: "swift"))
let postDescription = firstPost.description()
Matthew Spear
14,534 PointsMatthew Spear
14,534 PointsLook at this after you've attempted it (more likely to learn that way):
So there are two options here either passing in a variable of type Tag (1) or creating a Tag instance whilst creating the Post Struct (2):
(1):
(2):
let firstPost = Post(title: "Treehouse", author: "Pasan", tag: Tag(name: "Tag X"))
Either are of which are valid!