Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial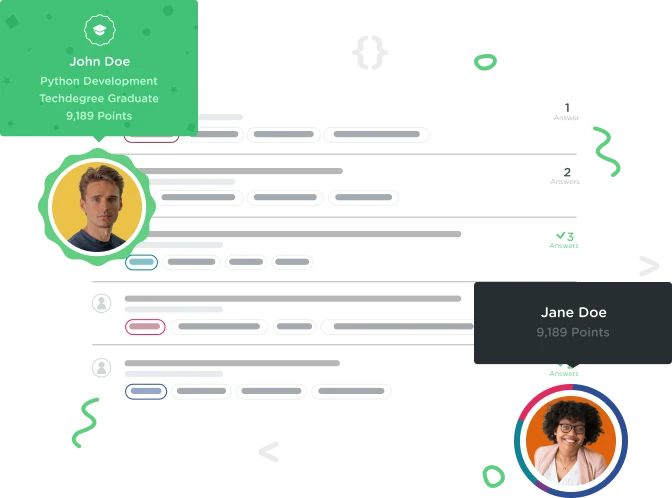

Matt Conway
1,572 PointsInstance for subclass?
Not sure about this ...
Challenge Task 1 of 1
In the editor, I have provided a class named Vehicle.
Your task is to create a subclass of Vehicle, named Car, that adds an additional stored property numberOfSeats of type Int with a default value of 4.
Once you've implemented the Car class, create an instance and assign it to a constant named someCar.
/Users/mattconway/Desktop/Screen Shot 2016-01-26 at 2.42.16 PM.png
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int
init(numberOfSeats: Int) {
self.numberOfSeats = 4
}
}
let someCars = Cars(numberOfSeats: 4)
4 Answers
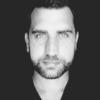
Jhoan Arango
14,575 PointsHello:
You are doing great, the only thing is that you do not have to provide it with an init method, since it's already inheriting one from the super class. Just give the store property a default value of 4. And when creating the instance of it, then you will use the init from the base class.
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int = 4
}
let someCar = Car(withDoors: 2, andWheels: 4)
Hope that helps.

Matt Conway
1,572 PointsI removed the init from Car class, but kept self.numberOfSeats and it still doesn't work. Made it worse, more errors now. Not sure???
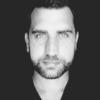
Jhoan Arango
14,575 PointsLook at the answer again... Compare your code with the answer.
self.numberOfSeats should be removed. You only should add the store property and give it initial values or otherwise known as hardcoded values to it.
var numberOfSeats: Int = 4
Good luck

Matt Conway
1,572 PointsGot the got that part. Trying to create an instance, "let someCar = Car()", it doesn't work. Neither does someCar.Car(). Actually I'm not really sure about the difference between the two.
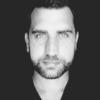
Jhoan Arango
14,575 PointsYou have to give values to the store properties through the init method.
Your Car class is inheriting from Vehicle class.. Vehicle class has an init method already implemented, therefore when you try to create an instance of "Car", you will be able to use the init it's inheriting from "Vehicle"
let someCar = Car(withDoors: 2, andWheels: 4)
See how (withDoors: Int, andWheels: Int) is implemented in the "Vehicle" class, but yet we are using it in the "Car" instance? That's because it's inheriting from it.
On my first answer, I gave you the whole code to the challenge, you can look at it, and compare it to yours and see what you are doing wrong.

Matt Conway
1,572 PointsOk, got it all now. Thanks for clear explanations.

Daniel Aunspach
942 PointsJhoan and Matt, thanks for posting the question and answer. I ran into trouble, as well along the same lines in that I also tried initializing the subclass (as it seemed to me the instructor advised in the lesson on overriding properties). Perhaps this code challenge should have been placed between the Inheritance and Overriding Properties videos?
Matt Conway
1,572 PointsMatt Conway
1,572 PointsOops, I added an "s" to "someCar" and "Car" in the let statement. Still doesn't work in Playground and Challenge without the "s".