Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial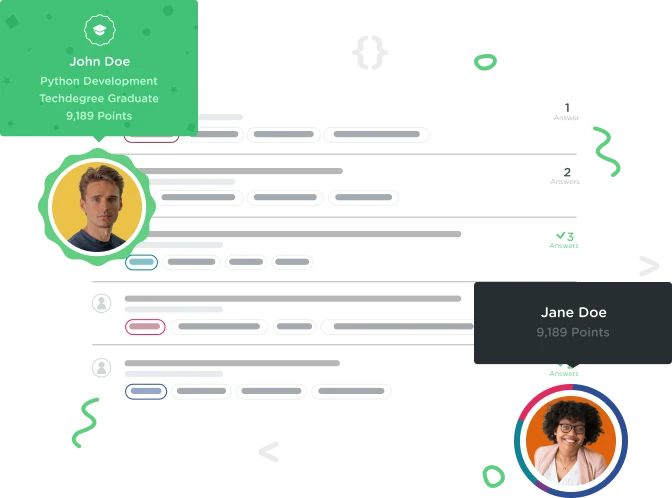

Julia Fitzer
Front End Web Development Techdegree Student 5,574 Pointsinstance variables within a method
Let's say you define a method within a class and you want to use an instance variable. Must you always include the @ ? That doesn't seem to be the case based on a quiz answer I gave that "passed". Or is this a matter of convention and good style?
For example, let's say the class has the instance variable @title and I want to write a method to return the title, both of these seem to work:
def title
title
end
def title
@title
end
1 Answer
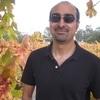
Kourosh Raeen
23,733 PointsHi Julia - Instance variables do need to start with @, otherwise they would be considered a local variable. I don't think the example you mentioned would work. This is what I tried:
class Book
def initialize(author, title)
@author = author
@title = title
end
def title
@title
end
end
book = Book.new("David A. Black", "The Well-Grounded Rubyist")
puts book.title
The above code works and the title method returns the value of the instance variable @title. Now try this:
class Book
def initialize(author, title)
@author = author
@title = title
end
def title
title
end
end
book = Book.new("David A. Black", "The Well-Grounded Rubyist")
puts book.title
This time you would get the error "Stack level too deep" because ruby thinks that the method title is calling itself, creating a recursion that will never stop.
Now, lets add another variable insdie the initialize method but this time without the @ symbol:
class Book
def initialize(author, title)
@author = author
@title = title
number_of_page = 538
end
def title
@title
end
def pages
number_of_page
end
end
book = Book.new("David A. Black", "The Well-Grounded Rubyist")
puts book.title
puts book.pages
This time you get the error "undefined local variable or method number_of_pages", because number_of_pages is a local variable that is only accessible inside the initialize method not anywhere else. Ok, lets now try this:
class Book
def initialize(author, title)
@author = author
@title = title
end
def title
@title
end
def pages
number_of_page = 538
return "This book has #{number_of_page} pages."
end
end
book = Book.new("David A. Black", "The Well-Grounded Rubyist")
puts book.title
puts book.pages
This time it works since we are using the local variable number_of_pages in the method where it is defined.
Hope this helps and happy coding!