Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial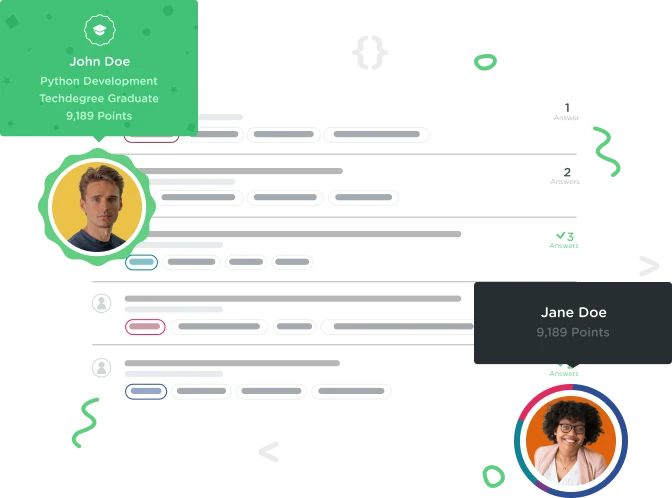

Heather Mathewson
10,912 Pointsinstances
In the editor the protocol you created, UserType, is provided along with an empty struct. For this task, Person needs to conform to UserType. After you've written code to achieve this task, create an instance of Person and assign it to a constant named somePerson.
I can figure out how to do the first step, but when it wants me to create an instance of Person and assign it to a constant named somePerson I get stuck.
protocol UserType {
var name: String { get }
var age: Int { get set }
}
struct Person: UserType {
var name: String
var age: Int
}
let person = Person
let somePerson = Person.person
1 Answer
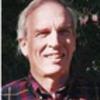
jcorum
71,830 PointsHeather, to create an instance of Person you do this:
let somePerson = Person(name: "x", age: 12)
What this does is call the default initializer for the Person struct and gives it values for each of its parameters. Note that the parameters have to be named name and age.
It might help if you saw the default initializer If you were to put it in the struct the struct would look like this:
struct Person: UserType {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
let somePerson = Person(name: "x", age: 12)
And it will work just like the code without it. When you type Person(name: "x", age" 12) you are calling this initializer with the two values it is expecting. The initializer creates two local variables named name and age, fills them with the values you supplied, and then it assigns those values to its member variables. So name in the somePerson Person object becomes "x", and age becomes 12.
If you write this in Treehouse's Challenge workspace
console.log(somePerson.name)
or this in a Playground
print(somePerson.name)
you would get x. If you have Xcode, I suggest you copy this, create a new Playground, paste it in, and play with it.
Heather Mathewson
10,912 PointsHeather Mathewson
10,912 PointsThank you so much! I had gotten really close to that, but then tried things just slightly off, which led to a weird road.
Andrew Taylor
11,500 PointsAndrew Taylor
11,500 PointsDo you need to do an init as it's a Struct not a clasS?