Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial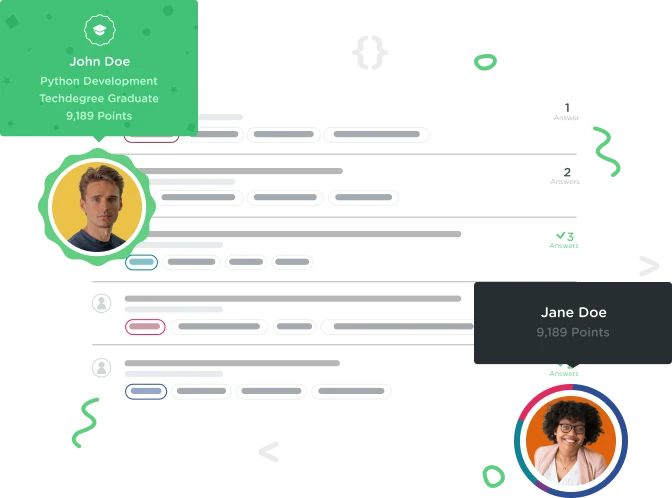

Justin Ezor
2,193 PointsInstead of viewing this URL in the browser, change the Intent to view it using the WebViewActivity class
Here's my code:
''import android.os.Bundle; import android.content.Intent; import android.net.Uri; import android.webkit.WebView;
public class WebViewActivity extends Activity {
protected String mUrl;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_web_view);
WebView webView = (WebView) findViewById(R.id.webView1);
Intent intent = new Intent(this, WebViewActivity.class);
intent.setData(Uri.parse(mUrl));
startActivity(intent);
}
}''
Here's the error I get:" Make sure you use the Intent constructor that takes two parameters! The first is the current context and the second is the target class."
I feel like I'm close! I tried searching Stack Overflow and google in general but am struggling to find anything helpful... Please help! :)
7 Answers
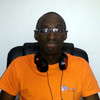
Alexandre Juca
Courses Plus Student 3,222 PointsHere is the code you need: @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_web_view);
WebView webView = (WebView) findViewById(R.id.webView1);
Intent intent = getIntent();//Here you are retrieving the intent you created and storing in the reference variable intent. You can also store extra info in the intent and pass it on.This way you can pass data from one Intent to another.
}
: ) Have fun coding!
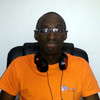
Alexandre Juca
Courses Plus Student 3,222 PointsFrom the above code I can see that your current context is this or the class WebViewActivity and the activity your trying to start is also the WebViewActivity class. The second argument should be another Activity.

poltexious
Courses Plus Student 6,993 PointsHi everyone,
I do not understand why people here cannot use Markdown code properly in this post? No offense, but it was not easy for me to find the answer among all of the answers. My point is that it can be hard to spot the answer if the users do not use the Markdown code the "right way".
Another thing I do not get is why the "Best Answer" goes to Alexandre Juca. His answer has nothing to do with the exact first task why selecting his answer as the "Best Answer" is totally misleading!
I have decided to post an answer in case a future reader needs help, and needs an answer that is easy to spot. The simple answer to this task is to ignore the:
// Add code here!
on line 17 in the WebViewActivity.java tab, which I got confused about, and simply modify line 25 (in my case) in the CustomListActivity.java tab from:
Intent intent = new Intent(Intent.ACTION_VIEW);
to:
Intent intent = new Intent(this, WebViewActivity.class);
Again, please do not get mad about this post. I just wanted to clear up things a little more.
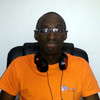
Alexandre Juca
Courses Plus Student 3,222 PointsIt should be like this Intent intent = new Intent(this, WebViewPageView.class);
1st arg(this is the current context) 2nd arg(The class or Activity responsible for displaying the webview)

Justin Ezor
2,193 PointsI responded as another answer look below

Justin Ezor
2,193 PointsHey Alexandre,
Thanks for the response! :) Unfortunately, I tried implementing what you told me and it didn't work out. I got this error: "Bummer! There is a compiler error. Please click on preview to view your syntax errors!"
The Preview window tells me this: "./WebViewActivity.java:16: cannot find symbol symbol : class WebViewPageView location: class WebViewActivity Intent intent = new Intent(this, WebViewPageView.class); ^ 1 error"
Here's my code with your reccommendations:
''import android.os.Bundle; import android.content.Intent; import android.net.Uri; import android.webkit.WebView;
public class WebViewActivity extends Activity {
protected String mUrl;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_web_view);
WebView webView = (WebView) findViewById(R.id.webView1);
Intent intent = new Intent(this, WebViewPageView.class);
intent.setData(Uri.parse(mUrl));
startActivity(intent);
}
}''

Sam Bass
9,038 PointsDid someone find the solution of this challenge? I can't even pass the 1 part of it :/

Justin Ezor
2,193 PointsHey Sam Bass I ended up getting help from a developer friend.
He referenced me this link to the Android Developer's guide: http://developer.android.com/training/basics/firstapp/starting-activity.html
Check out the "Build an Intent" section. That should point you in the right direction!

Andy Sing
2,190 PointsIntent intent = new Intent(this, WebViewActivity.class);
also you are modifying code not completely adding