Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial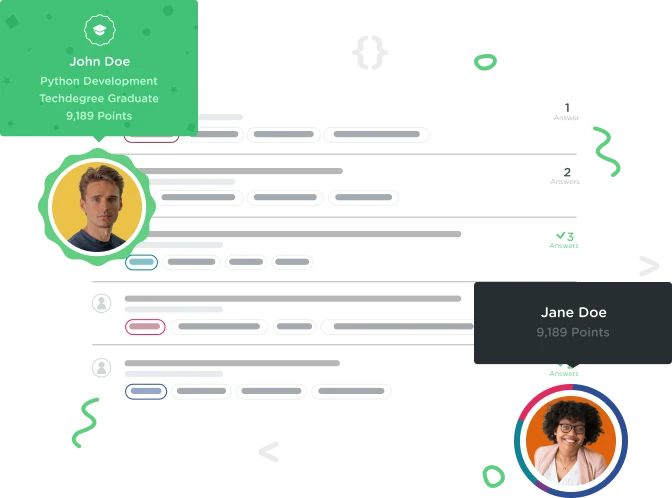

Alejandro Garcia
2,103 PointsInstructions are not very clear. Can someone explain?
What is it really asking? Create a do while where the answer is always no?
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
boolean answer;
do{
console.readLine("Do you understand do while loops?");
answer = "no";
}
while{
consle.readLine("Do you understand do while loops?");
}
2 Answers

poltexious
Courses Plus Student 6,993 PointsThere are 3 tasks in this code challenge.
Task 1: Prompt the user with the question "Do you understand do while loops?". Store the result in a new String variable named response.
The answer is as follows:
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
response = console.readLine("Do you understand do while loops?");
Here you declare a String object, and initialise it with the console.readLine prompting with the question. The user then types in something which will be stored in the response String object.
Task 2: Now continually prompt the user in a do while loop. The loop should continue running as long as the response is No. Don't forget to declare response outside of the do while loop.
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
do {
response = console.readLine("Do you understand do while loops?");
}
while(response.equalsIgnoreCase("No"));
Here you simply have to declare the do while loop as above. The thing here is that while response.equalsIgnoreCase("No") is true, the code in the do section will continue repeating until the response is actually NOT "no" (which should not be case sensitive, and that is why I use the equalsIgnoreCase() method).
Task 3: Finally, using console.printf print out a formatted string that says "Because you said [response], you passed the test!"
So here I simply add one line of code in the end of the do section:
console.printf("Because you said %s, you passed the test!", response);
The reason why I use %s with the response String object in that line of code is because whatever the response is (and it is not no), it will be printed out as a message with the user's stored response.
The final code will look like this:
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
do {
response = console.readLine("Do you understand do while loops?");
console.printf("Because you said %s, you passed the test!", response);
}
while(response.equalsIgnoreCase("No"));
I hope it makes sense now.

poltexious
Courses Plus Student 6,993 PointsBy the way... you are making some errors in your code by using the { } brackets in the while section instead of ( ) brackets.
Also, consle should be console, and your boolean answer is not really necessary here.

Kenneth Balajadia
2,009 PointsThanks poltexious
poltexious
Courses Plus Student 6,993 Pointspoltexious
Courses Plus Student 6,993 PointsBest answer would be appreciated :-)