Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial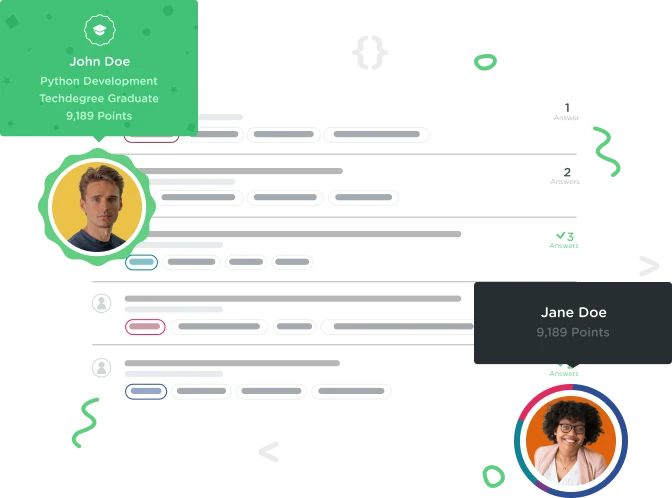

Josiah McClellan
Courses Plus Student 7,869 PointsInstructor made a mistake. Naming a function doesn't always assign it to a variable automatically.
The instructor had the following code on the screen:
callTwice(function namedFunction(){
//some arbitrary example code
});
And he said the following words:
"However, this does create the variable called namedFunction, which is accessible from the global scope, so really what this is doing is it's exactly the same as declaring a variable and passing it in."
Correct me if I'm wrong, but I think that is simply untrue. I tried this code myself in the console to be sure, and it went like this:
> function callTwice(f){f();f();}
undefined
> callTwice(function namedFunction(){});
undefined
> namedFunction()
VM109:2 Uncaught ReferenceError: namedFunction is not defined
I wish I understood the exact rules well enough to say exactly when function namedFunction(){...}
does create a variable, but I do know that this is not one of those times.
1 Answer
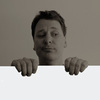
Sean T. Unwin
28,690 PointsVery interesting question.
Scope here, I think, was probably the incorrect word to use. The JavaScript interpreter or engine has access to it and knows it's name and can therefore output it's name, which seems obvious and it likely is, but I think that is what was meant in order to get the point across that anonymous functions have no name. If you add console.log(f);
to callTwice
so it looks like:
function callTwice(f){
f();
f();
console.log(f);
}
You will see that 'namedFunction' is output.
However, this doesn't mean it's available in the global scope in the sense that is typically meant where other code can reference it at anytime. This is an example of a "function expression" and they are not visible outside of it's scope. In this particular case, the scope is the function it was declared in, and I suppose more specifically within the arguments
of the function. So it is, in fact, a variable as an item within the arguments
array. Since it is in the arguments
array, garbage collection is done as soon as the function is finished executing which means there will be no reference to it afterwards. So namedFunction
can be utilized, and known, anywhere within callTwice
, but ONLY within that function call where it is declared, since it's passed as an argument and created at that point.
I likely didn't answer that as well as intended, yet I hope that I helped make the concept a little clearer to understand.