Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial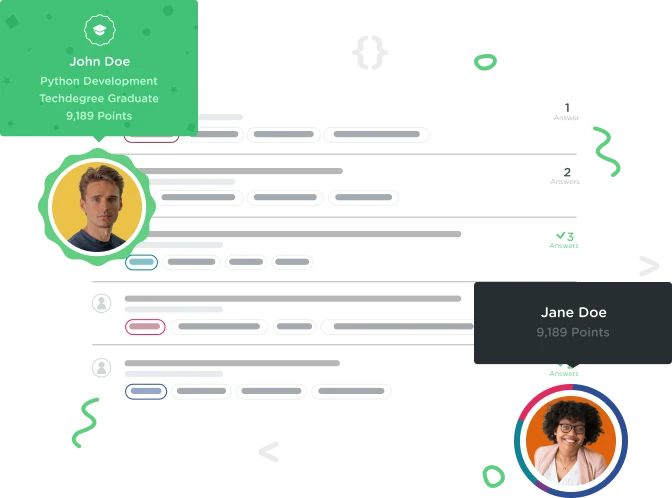

Kenneth Dubroff
10,612 PointsInt compared to Bool
in the fizzbuzz challenge, I'm using a comparative operator to check for a remainder of 0. When I do this, swift returns a Bool, but an Int is expected in order to compile. I get something like an Int can't be matched to a Bool
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n {
case n % 3 == 0: return "Fizz"
case n % 5 == 0: return "Buzz"
}
// End code
return "\(n)"
}
5 Answers
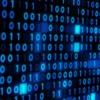
Alexander Davison
65,469 PointsYou can remove the n
in the switch (n) {
line of code, so that your code looks like this:
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch {
case n % 3 == 0: return "Fizz"
case n % 5 == 0: return "Buzz"
}
// End code
return "\(n)"
}
Just note that I am not 100% familiar with Swift, but at least in Ruby you are allowed to do this. If this method doesn't work, you can use an if/else if/else
clause.
I hope this helps
~Alex
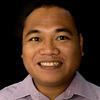
Mel John del Barrio
iOS Development Techdegree Student 8,484 PointsTry using the where clause for the conditions that needs to match with your n
let yetAnotherPoint = (1, -1)
switch yetAnotherPoint {
case let (x, y) where x == y:
print("(\(x), \(y)) is on the line x == y")
case let (x, y) where x == -y:
print("(\(x), \(y)) is on the line x == -y")
case let (x, y):
print("(\(x), \(y)) is just some arbitrary point")
}
// Prints "(1, -1) is on the line x == -y"

Kenneth Dubroff
10,612 PointsAlex, I'd love to use if/then statements since I understand the syntax perfectly well. However, this challenge is specifically to use switch. Switch can accept arguments and is much more powerful than if/else. The syntax is just different

Kenneth Dubroff
10,612 PointsMel John del Barrio hit the nail on the head with syntax. That said, though it compiles, the challenge doesn't accept my answer.
Am I correct in that n %3 == 0 should be checking if n is divisible by 3?
my code looks like this:
switch n {
case let n where n % 3 == 0: return "Fizz"
case let n where n % 5 == 0: return "Buzz"
case let n where n % 3 == 0 && n % 5 == 0: return: "FizzBuzz"
}
I had to enter a default case in order for the compiler to accept the switch statement, though the challenge says not to enter a default case

Kenneth Dubroff
10,612 PointsAccepted answer: (precedence matters!) The compiler stopped at let n % 3 == 0 and didn't check if the number was divisible by both 3 and 5 because the condition was satisfied. I had to move the line checking for both 3 and 5 to the top. Also, though the challenge specifically asks not to enter a default case, one MUST be entered.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n {
case let n where n % 3 == 0 && n % 5 == 0: return "FizzBuzz"
case let n where n % 3 == 0: return "Fizz"
case let n where n % 5 == 0: return "Buzz"
default: return "\(n)"
}
// End code
return "\(n)"
}

Kenneth Dubroff
10,612 PointsHard to pick a best answer here. Alex's answer of using if/else is actually correct, and the most common solution. However, the code I used (Mel John del Barrio's example) performs the same function, and uses less lines of code. It's also less characters per line of code, leaving less room for syntax/spelling errors.
In my defense, the lesson went over switch vs if/else and specifically stated that switch is basically better (more powerful). Using a combination of if/else and switch seems clunky and unnecessary
At any rate, thanks to both of you. Not sure if a mod will pick best answer since I'm undecided, but may the victor take the spoils!
Kenneth Dubroff
10,612 PointsKenneth Dubroff
10,612 PointsThanks for the input, Alex.
That doesn't work in Swift, as an argument is required after switch. It also doesn't do anything to address the Int/Bool issue
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsSorry for not explaining
I was kinda lazy on the answer
Anyway, you shouldn't use a
switch
statement for this challenge.You use the
switch
statement like this:In this code...
As you see, you don't need a condition for the
case
s. It automatically checks if the focused variable (in my codea
) is the value aftercase
. In Ruby, you can specify no variable to focus on and enter conditions after eachcase
statement instead of a number. Since I'm unfamiliar with Swift, I'm not sure if you can do this.Use a
if/else if/else
condition instead.EDITED MAJOR INFORMATION, PLEASE RE-READ