Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial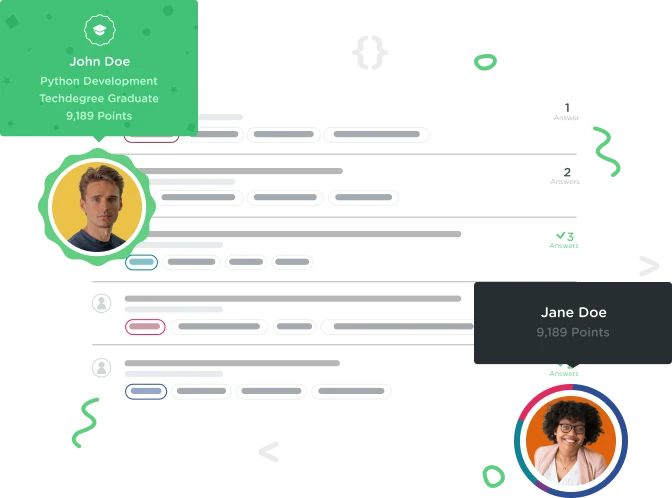

Enes artun
Courses Plus Student 2,364 Points'int' object has no attribute 'columns'
While studying game class part 2, I am getting this error. What should I change here?
'''python
from cards import Card import random
class game:
def __init__(self):
self.size = 4
self.card_options = ['Add', 'Boo', 'Cat', 'Dev',
'Egg', 'Far', 'Gum',]
self.columns = ['A', 'B', 'C', 'D']
self.cards = []
self.locations =[]
for column in self.columns:
for num in range(1, self.size +1):
self.locations.append(f'{column}{num}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for columns in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row '''
''' if name == 'main': game1 = game() game1.set_cards() game1.cards[0].matched = True game1.cards[1].matched = True game1.cards[2].matched = True game1.cards[3].matched = True print(game.create_row(1, 1)) print(game.create_row(2, 2)) print(game.create_row(3, 3)) print(game.create_row(4, 4)) '''
for columns in self.columns:
AttributeError: 'int' object has no attribute 'columns'
1 Answer
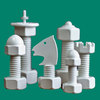
Steven Parker
231,269 PointsIn the main code (the part not formatted above), the method "create_row" is being called directly on the class "game" instead of the object "game1". This causes the error message you see.
Also, that method is being called with two numeric arguments, but it is defined to take only one.
Additionally, the loop variable in the "create_row" method is "columns" (plural) but the print statement refers to "column" (singular).
Steven Parker
231,269 PointsSteven Parker
231,269 PointsWhen posting code, use Markdown formatting to preserve the code's appearance. This is particularly important with Python where indentation controls program flow. Be aware that the symbols that indicate a Markdown code block are accents (```), not apostrophes (''').
And an even better way to show code is by sharing a snapshot of your workspace.