Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial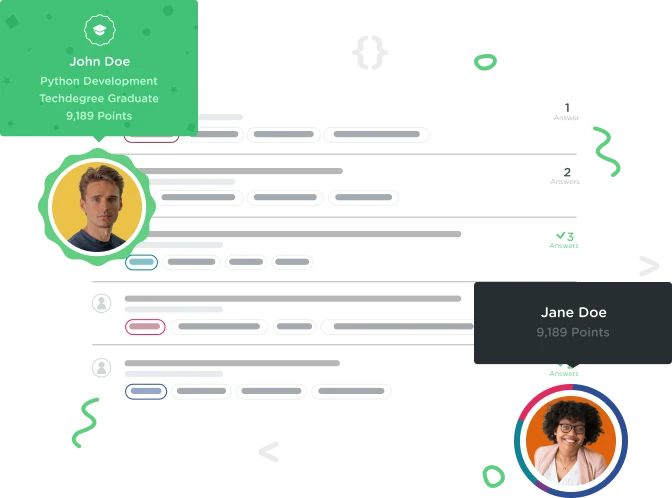

John Jordan
880 PointsIntegers for index
It is telling me that I don't have integer indexes, but I used '//' I also tried this with the 'int()' function. Where is my error?
Thanks
def sillycase(word):
halfpoint = len(word)//2
word[halfpoint:] = word[halfpoint:].upper()
word[:halfpoint] = word[:halfpoint].lower()
return word
2 Answers

andren
28,558 PointsThat is actually a generic error message that the challenge gives whenever your code does not work properly. The actual issue with your code is the fact that you try to modify the contents of the word
string. Strings are immutable (meaning you cannot directly change them) so trying to modify the contents of the string like you are doing in your code is not valid.
If you either use separate variables to hold the two parts and then concatenate them like this:
def sillycase(word):
halfpoint = len(word)//2
upper_half = word[halfpoint:].upper()
lower_half = word[:halfpoint].lower()
return lower_half + upper_half
Or just concatenate the slices directly like this:
def sillycase(word):
halfpoint = len(word)//2
return word[:halfpoint].lower() + word[halfpoint:].upper()
Then your code will pass.

John Jordan
880 PointsInteresting distinction between lists and strings. Thank you