Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial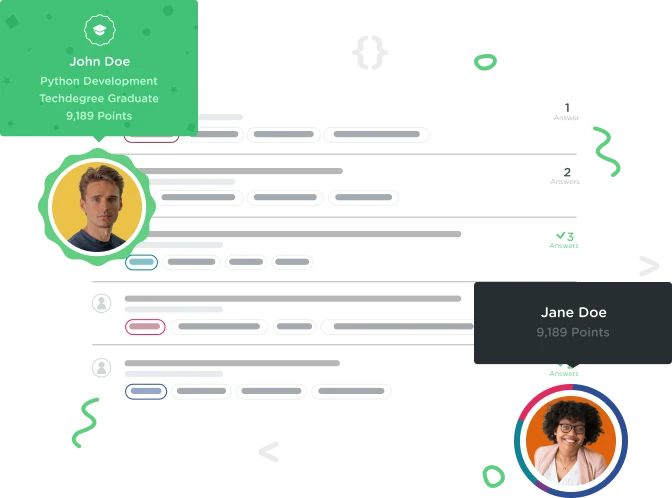
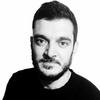
Filip Grkinic
7,610 PointsIntegrating Validation Errors - Extra Credit
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = trim($_POST["name"]);
$email = trim($_POST["email"]);
$message = trim($_POST["message"]);
$error_message = array();
// 1. check if required fields are entered
if ($name == "" OR $email == "" OR $message == "") {
array_push($error_message, "You must specify a value for name, email and message.");
}
// 2. check if fields have malicious code and if $error_message is NOT set from 1. check
if (!isset($error_message)) {
foreach ($_POST as $value) {
if (stripos($value, 'Content-Type:') !== FALSE) {
array_push($error_message, "There was a problem with the information you entered");
}
}
}
// 3. check if hidden address input is left blank and if $error_message is already filled with 1. or 2.
if (!isset($error_message) && $_POST["address"] != "") {
array_push($error_message, "Your form submission has an error.");
}
require_once("inc/phpmailer/class.phpmailer.php");
$mail = new PHPMailer();
// 4. check if email address is a valid format
if (!isset($error_message) && !$mail->ValidateAddress($email)) {
array_push($error_message, "You must specify a valid email address.");
}
//To-Do send email
if (!isset($error_message)) {
$email_body = "";
$email_body = $email_body . "Name: " . $name . "<br>";
$email_body = $email_body . "Email: " . $email . "<br>";
$email_body = $email_body . "Message: " . $message;
$mail->SetFrom($email, $name);
$address = "m4dcro@gmail.com";
$mail->AddAddress($address, "Shirts 4 Mike");
$mail->Subject = "Shirts 4 Mike Contact Form Submission | " . $name;
$mail->MsgHTML($email_body);
if ($mail->Send()) {
header("Location: contact.php?status=thanks");
exit;
} else {
array_push($error_message, "There was a problem sending the email: " . $mail->ErrorInfo);
}
}
}
?><?php
$pageTitle = "Contact Mike";
$section = "contact";
include('inc/header.php'); ?>
<div class="section page">
<div class="wrapper">
<h1>Contact</h1>
<?php
if(isset($_GET["status"]) AND $_GET["status"]=="thanks"){ ?>
<p>Thanks for the message!</p>
<?php } else { ?>
<?php
if (!isset($error_message)) {
echo '<p>Love to hear from you! Complete the form to send me an email.</p>';
}
foreach ($error_message as $error) {
echo '<p class="message">' . $error . '</p><br>';
exit;
}
?>
<form action="contact.php" method="post">
<table>
<tr>
<th>
<label for="name">Name </label>
</th>
<td>
<input type="text" name="name" id="name" value="<?php if (isset($name)) {echo
htmlspecialchars($name);} ?>"/>
</td>
</tr>
<tr>
<th>
<label for="email">Email </label>
</th>
<td>
<input type="text" name="email" id="email" value="<?php if (isset($email)) {echo
htmlspecialchars($email);} ?>"/>
</td>
</tr>
<tr>
<th>
<label for="name">Message </label>
</th>
<td>
<textarea name="message" id="message"><?php if (isset($message)) { echo
htmlspecialchars($message); } ?>
</textarea>
</td>
</tr>
<tr style="display:none;">
<th>
<label for="address">Address</label>
</th>
<td>
<input type="text" name="address" id="address">
<p>Humans: please leave this field blank.</p>
</td>
</tr>
</table>
<input type="submit" value="Send"/>
</form>
<?php } ?>
</div>
</div>
<?php include('inc/footer.php'); ?>
what am I doing wrong? Thanksss!
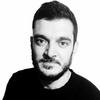
Filip Grkinic
7,610 PointsYou need to read what is wanted in the extra credit from that particular task. So I'm using foreach so I can echo all errors if there are more for the form. Right now, it doesnt echo all errors, but it outputs nothing.
Im not sure where should I put foreach loop.
2 Answers
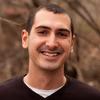
tihomirvelev
14,109 PointsThe foreach loop is at the right place. The problem is that with the creation of the $error_message array, the !isset check will always give a false result and this is breaking things up.
The solution is to remove all !isset($error_message) and leave the other conditions for check.
For example, #3 if block has to look that way.
if ($_POST["address"] != "") {
array_push($error_message, "Your form submission has an error.");
}
I hope you understood me.
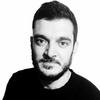
Filip Grkinic
7,610 Pointsgreat, that makes sense. Thank you. Since I created an array, its already set.. so theres no point in checking with isset function.
In the last conditional that actually sends the email is it ok to use if(empty($error_message) instead of !isset($error_message) ?
Because if the array is empty, proceed, if its not, echo all errors through foreach loop.
edit: it works! :)) https://www.dropbox.com/s/1gy2wrcvg2w54rh/Screenshot%202014-07-29%2011.31.51.png
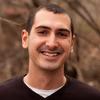
tihomirvelev
14,109 PointsYes, that's right. Before sending the email you want to check if there were any errors in $error_message. If there are errors and the array is not empty, then you won't send the email and you'll output all errors.
So if(empty($error_message)) will work great.
tihomirvelev
14,109 Pointstihomirvelev
14,109 PointsAt first glance I don't see the problem. Can you describe what is the problem you are trying to fix? It there some type of error or...?