Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial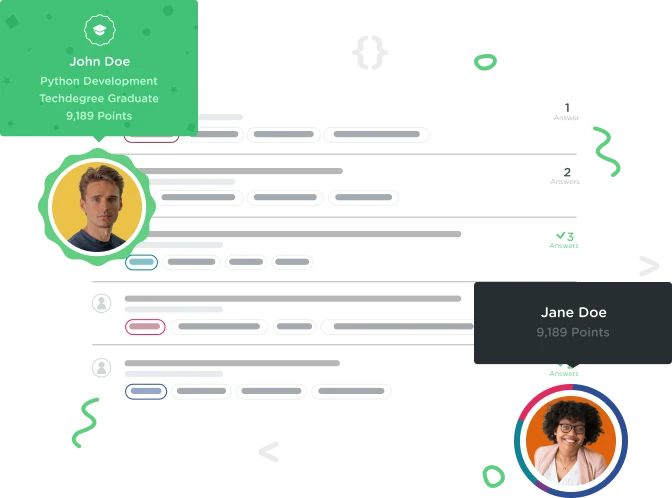

ihssan hashem
2,730 PointsIntents
Hi, so I'm doing the extra credits and I have two questions :D
1: I want to pass an Object through intent, is that possible? I searched and found that I need to convert the object class into a parcelable class, but I was confused and couldn't do it.
2: my other question is that I have 3 activities, I'm passing a text from the first activity to the second using an intent, and I want to pass another string from the second activity to the third using putExtra, the first putExtra is working fine, but the second one just doesn't receive the sent data. Is it getting confused with the previous intent? is their any solution to this problem?
Thanks :D
2 Answers
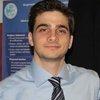
adamabdulghani
7,864 PointsHi Ihssan,
Concerning your first question, a more simple solution than using Parcelable is to implement Serializable on your Class, this way you could use [this] http://developer.android.com/reference/android/content/Intent.html#putExtra(java.lang.String, java.io.Serializable)
intent.putExtra("myObject", myObject);
// And to retrieve the object you could
getSerializableExtra("myObject");
For your second question, can you share your code probably here or on Github?
Thanks :)

ihssan hashem
2,730 PointsThanks a lot man, it's all working now and I was able to move the whole object :D feels great :) Thanks!
ihssan hashem
2,730 Pointsihssan hashem
2,730 PointsThanks for responding :) I tried the serializablation, but it's not working probably because of the same problem I asked about in the second question.
Here I'm sending the object from the first activity:
Here i'm getting the intent from the other activity I tried the same thing with sending texts through the intent and it didn't work too, it is not receiving any texts the string becomes empty.
adamabdulghani
7,864 Pointsadamabdulghani
7,864 PointsFantastic! you just need to cast to Serializable when you putExtra, and make sure your User class implements Serializable.
Hope this helps :)