Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial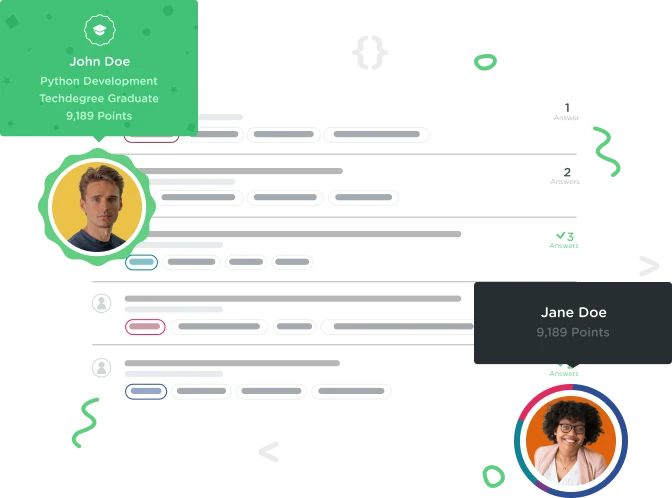
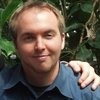
Eric Conklin
8,350 PointsInteractive pages with Javascript
The below code does not produce the desired results. When I click the check box on a to do item, it goes to the task completed list, but the inverse does not happen when I click the task completed list.
It is supposed to remove it from the completed list and take it back to the regular list, but this does not happen.
Any help would be appreciated.
//Problem: User interaction doesn't provide desired results
//Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //New task
var addButton = document.getElementsByTagName("button")[0]; //First button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //#incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //#completed-tasks
//New Task List Item
var createNewTaskElement = function(taskstring){
//Create List Item
var listItem = document.createElement("li");
//input checkbox
var checkBox = document.createElement("input"); //checkbox
//label
var label = document.createElement("label"); //label
//input text
var editInput = document.createElement("input"); //text
//button.edit
var editButton = document.createElement("button"); //edit
//buton.delete
var deleteButton = document.createElement("button"); //delete
//Each element needs to be modified
//Each element needs to be appended
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
}
//Add a new task
var addTask = function(){
console.log("Add task...");
//When the button is pressed
//Create a new list item with the text from the #new-task:
var listItem = createNewTaskElement("Some new task");
//Append listItem to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Edit an existing task
var editTask = function(){
console.log("Edit task...");
//When the edit button is pressed
//If the class of the parent is .editMode
//Switch from .editMode
//label text become the input's value
//else
//Switch to .editMode
//input value becomes to label's text
//Toggle .editMode
}
//Delete an existing task
var deleteTask = function(){
console.log("Delete task...");
//When the delete button is pressed
//Remove the parent list item from the ul
}
//Mark a task as complete
var taskCompleted = function(){
console.log("Complete task...");
//Append the task list item to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Mark a task as incomplete
var taskinComplete = function(){
console.log("Task incomplete...");
//Append the task list item to the #incomplete-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
var bindTaskEvents = function(taskListItem, checkboxEventHandler){
console.log("Bind list item events");
//select taskListItem's children
var checkbox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind taskCompleted to checkbox
checkbox.onchange = checkboxEventHandler;
}
//cycle over incompleteTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++){
//bind events to list items children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over TasksHolder ul list items
for(var i = 0; i < completedTasksHolder.children.length; i++){
//bind events to list items children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskCompleted);
}
2 Answers
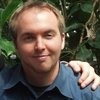
Eric Conklin
8,350 PointsFixed it. Changed the taskholder code at the bottom to the following code:
//cycle over TasksHolder ul list items
for(var i = 0; i < completedTasksHolder.children.length; i++){
//bind events to list items children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskInComplete);
}
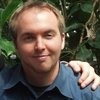
Eric Conklin
8,350 PointsThe issue was the confusing taskIncomplete and taskCompleted. Very confusing. Make sure they line up with which function you are using with the bindTaskEvents function.