Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial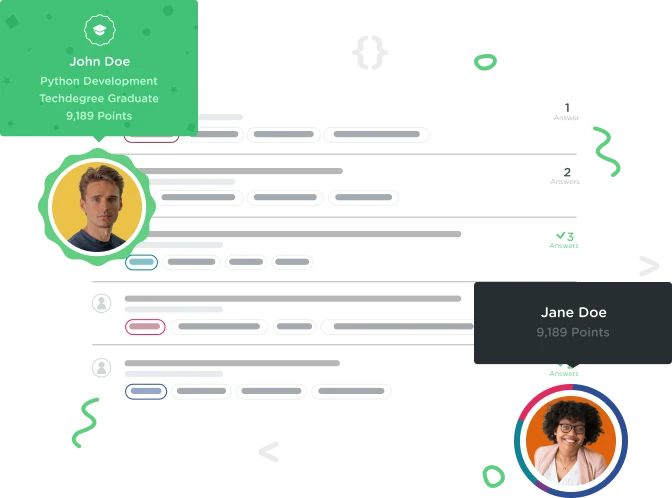
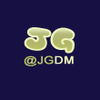
Jonathan Grieve
Treehouse Moderator 91,252 PointsInterfaces and Serialisation in Java. Am I getting this right?
Am I getting this right? I'm not completely sure on Serialization as it seems others not just me have struggled with this but I can hope at least I've got the theory right.
Implements
Interface - like a contract programmer signs when they add to the list of interfaces that the class implements.
It's a guarantee from the programmer. The programmer then has to work out how to keep that guarantee or program fails to compile.
"implements" are added to a class. You use the
implements
keyword and specify the name of the interface. A common example is Comparable.
public class NameofClass implements Comparable {
}
- You can check your code meets the "contract" by using instanceof.
Comparable
is a keyword that tells the class that it needs a certain method to compare one object to another and that it should return an integer. and that should return an integer that denotes whether it is less than, equal to, or greater than the object passed in as a parameter.
Comparable
does is tell every class that implements it that it has to have a compareTo
method that takes in another object of the same type, and that should return an integer that denotes whether it is less than, equal to, or greater than the object passed in as a parameter
So the code now is expecting the programmer to override an abstract method from Object, i.e. the compareTo() method. As far as I'm aware this is the only method you can do this with.
need to include a method called compareTo. The implements keyword is comparable so need to compare the method/definition.
From the documentation
int compareTo(T o)
T = the type of iobjects that this object may be compared to
o = object to be compared
It either returns a negative, zero or positive.
You can have multiple interfaces defined in a class (comma separated)
We use @override annotation in the same way as overriding a normal method. Here we're overriding an implementation.
Code Example
public int compareTo(Object obj) {
//write code
}
public int compareTo(Object obj) {
Treet other = (Treet) obj;
if (equal(other)) {
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if(dateCmp == 0) {
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
Interface lists - http://docs.oracle.com/javase/8/docs/api/
Serialization
Serialization - https://teamtreehouse.com/library/java-data-structures/organizing-data/serialization
is the process of translating objects into a format that can be stored and recreated.
Ever wanted to save an object for later after the program is done running. Well this is called serialization.
Serialization is taking an object and storing its representation in a state that can be loaded.
Serialization requires that the object implements the serialization interface which doesn't have any required methods.
It's a "marker" interface, an interface that is marked with an ability lijk the ability to be serialized.
import java.io.Serializable;
public class ClassName implements Comparable, Serializable. <--- class is now serializable.
Can run little methods on it like save();
Lakshmi Narayana Dasari
1,185 PointsLakshmi Narayana Dasari
1,185 Pointsperfect