Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial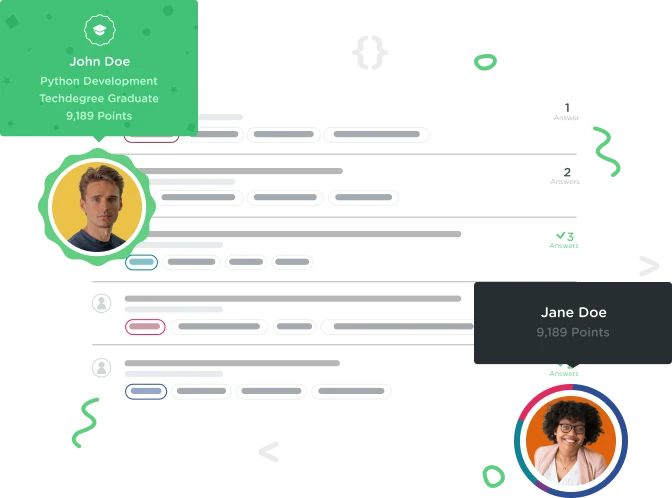

Bjorn Van de Weyngaert
1,297 PointsInterfaces: can't wrap my head around this line of code
I'm looking into the subject of interfaces. In the exercise I've written this line of code:
@Override
public int compareTo(Object obj) {
BlogPost other = (BlogPost) obj;
if (equals(other)) {
return 0;
}
return 1;
}
Question 1: So first we place the value for "obj" into "other" and then we ask if "obj" equals "other". Correct? But I read this code as if we made them equal in the line before that (as we stored "obj" in "other").
Question 2: In the prior video we had to write the same line of code for the "Treets" class. In Example.java we didn't use the compareTo method, but we did use the sort method for arrays. Does the sort method automatically calls the compareTo method? Otherwise, how can it know to sort by creationDate if we didn't call the compareTo method there?
I'm sure I'm overlooking something and as you see I'm pretty confused on this topic :-) Could someone please explain? Thanks.
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
@Override
public int compareTo(Object obj) {
BlogPost other = (BlogPost) obj;
if (equals(other)) {
return 0;
}
return 1;
}
}
1 Answer

andren
28,558 PointsSo first we place the value for "obj" into "other" and then we ask if "obj" equals "other". Correct?
The first part is pretty much correct. You take obj
and cast it down to a BlogPost
object and store it in other
. But you are misunderstanding the equals
line.
The equals
method belongs to the object that we are writing this method in. And it compares that object against the (other
) object that was passed in. That is the default behavior of the equals
method, which is a method that all object have since they inherit it from the Object
class.
So let's say we had code that looked like this: blogPost1.compareTo(blogPost2)
. In that case the equals
method would belong to and be comparing blogPost1
, and it would be comparing it against blogPost2
, which is what would be stored in the other
variable.
Does the sort method automatically calls the compareTo method?
Yes, it does. sort
only works on arrays of objects that implement the comparable
interface. And the reason for that is that it is depended on the objects having a compareTo
method for it to evaluate how it should sort the objects in the array.