Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial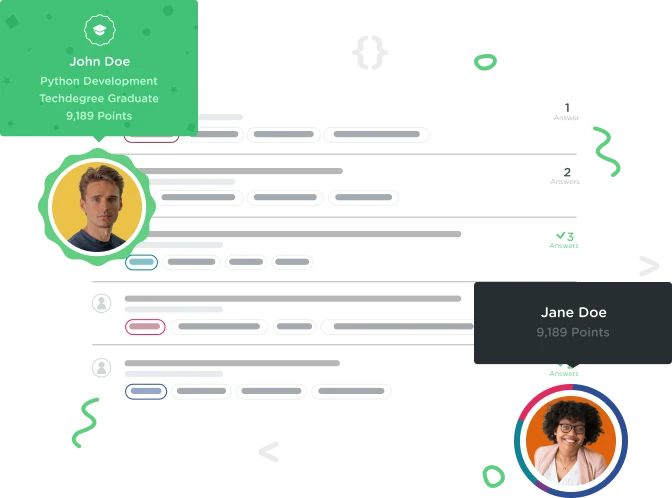
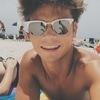
garyguermantokman
21,097 PointsIntermediate Swift 2 - Extensions
Anyone solve this code challenge. The solution I wrote works but It does not pass the challenge.
/*Challenge*/
extension String {
func addToString(number: Int) -> Int? {
switch self {
case Int.min.description ... Int.max.description:
return Int(self)! + number
default:
return nil
}
}
}
"66".addToString(5)
// Enter your code below
extension String {
func addToString(number: Int) -> Int? {
switch self {
case Int.min.description ... Int.max.description:
return Int(self)! + number
default:
return nil
}
}
}
2 Answers
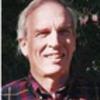
jcorum
71,830 PointsYes, I've found that the editor is picky, and often won't accept valid Swift code. It wants exactly what it asks for. Remember, your input is being checked by a program, not humans!
extension String {
func add(num: Int) -> Int? {
if let n = Int(self) {
return n + num
}
else {
return nil
}
}
}
var s = "2"
s.add(2)
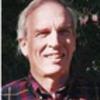
jcorum
71,830 PointsCody,
First, the challenge's example was "1".add(2), which hints it wants the method to be named add().
Second, the String (self) may or may not contain a number, so you need to test first to see if it can be converted to an Int. I used if let n = Int(self) to do the testing. If self is a String like "1" or "124" that can be converted to a number then n will have the converted value.
Note that you need to do this testing before you return anything, not during the return. Also note that self is a String, not an optional, so no unwrapping, forcible or not, is needed. We're not testing here if self is nil, we're testing if it's contents are a number or not: "2" vs "2B".
In this code:
var s = "2"
s.add(2) //4
s = "2B"
s.add(2) //nil
the second line will yield 4 and the 4th line nil.
Third, if n is an Int then you return n + num, where num is the value that is passed in to the add() function. Otherwise you return nil.
If any of this is puzzling, let me know and I'll try again.
Cody Adkins
7,260 PointsCody Adkins
7,260 PointsCan you break down your logic for me? I am trying to understand how it all works.