Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial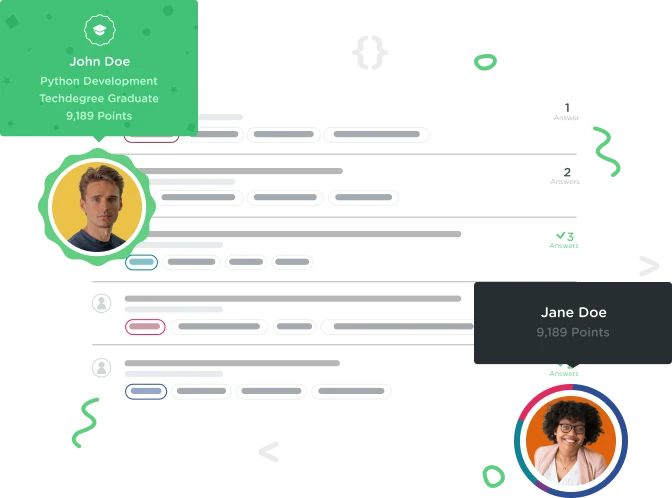

thomas2017
1,342 PointsIntro to number game for python. why else: doesn't run in my program?
I did mine differently than what the video showed. It would run but if I ran out of guesses then my else statement within my while loop doesn't get triggered. I want this else statement to get triggered so that it can call a function to tell the player they just lost and if they want to play again. I guess this is due to my elif stament right above it, but I don't know how else to qualify it.
https://gist.github.com/f8dd8f45dab3a2a7d9fe7ee
import random
def you_lose():
again = input("""sorry you ran out of chances.
type [y] play again [n] to quit.""")
if again.lower() == "y":
game()
else:
print("better luck next time")
def game():
chosen_num = random.randint(1, 10)
chances = 5
while chances > 0:
guess = int(input("Please enter a guess: "))
if guess == chosen_num:
print("you got it right")
play_again = input("you wanna play again buddy y/n?")
if play_again.lower() == "y":
game()
else:
print("Have a fabulous day")
break
elif guess != chosen_num and chances > 0:
chances -= 1
print("You have {} guess left.".format(chances))
if guess < chosen_num:
print("your number is higher than your guess. ")
elif guess > chosen_num:
print("your number is lower than your guess. ")
else:
you_lose()
game()```
3 Answers
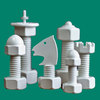
Steven Parker
231,275 PointsEven without proper formatting the ampersand (&) stands out in the "elif" line.
In Python, the logical AND operator is simply the word "and".

thomas2017
1,342 PointsHi Steven, I changed the ampersand sign out. I'm still getting the same result. If I can't guess the correctly within 5 chances it suddenly just stop. So I guess my question is why doesn't it get to the else statement right after this elif statement.

thomas2017
1,342 PointsHi Steven, so I got it to worked. I basicly moved all the code from the you_lose functions into the last else: block. So the problem was basicly calling on the you_lose function.
Here is my updated codes. import random
def you_lose():
again = input("""sorry you ran out of chances.
type [y] play again [n] to quit.""")
if again.lower() == "y":
game()
else:
print("better luck next time")
def game():
chosen_num = random.randint(1, 10)
chances = 5
while chances > 0:
try:
guess = int(input("Please enter a guess: "))
except ValueError:
print("{} isn't a number!".format(guess))
if guess == chosen_num:
print("you got it right")
play_again = input("""you wanna play again?...
you bedraggled indegene y/n?""")
if play_again.lower() == "y":
game()
else:
print("Have a bereft day!")
break
elif guess != chosen_num and chances > 0:
chances -= 1
print("You have {} guess left.".format(chances))
if guess < chosen_num:
print("your number is higher than your guess. ")
elif guess > chosen_num:
print("your number is lower than your guess. ")
###else:
##you_lose()
else:
again = input("""Hey my doughty friend...you're out of chances....
type [y] play again [n] to quit.""")
if again.lower() == "y":
game()
else:
print("Better luck next time.. epigone!")
game()```
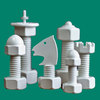
Steven Parker
231,275 PointsMuch better on the formatting! But be sure to put that last set of 3 back-ticks on their own line.
And congrats on the resolution.

thomas2017
1,342 PointsHi I still don't understand why I couldn't call the you close() function
######1st attempt and lose function couldn't get called####
##else:
##you_lose()
#####2nd attempt moving everything from you_lose() function down to the else: statement
##else:
again = input("""Hey my doughty friend...you're out of chances....
type [y] play again [n] to quit.""")
if again.lower() == "y":
game()
else:
print("Better luck next time.. epigone!")
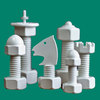
Steven Parker
231,275 PointsI'm not sure why you had difficulty. If I remove the comments and activate the function, it seems to work as expected:
import random
def you_lose():
again = input("""sorry you ran out of chances.
type [y] play again [n] to quit.""")
# <-- code in between omitted for brevity -->
elif guess > chosen_num:
print("your number is lower than your guess. ")
else:
you_lose()
# replicated function code removed
game()
Happy coding!
Steven Parker
231,275 PointsSteven Parker
231,275 PointsThat github link doesn't seem to work and Python really needs to be formatted to be readable.
Use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
 Or watch this video on code formatting.