Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial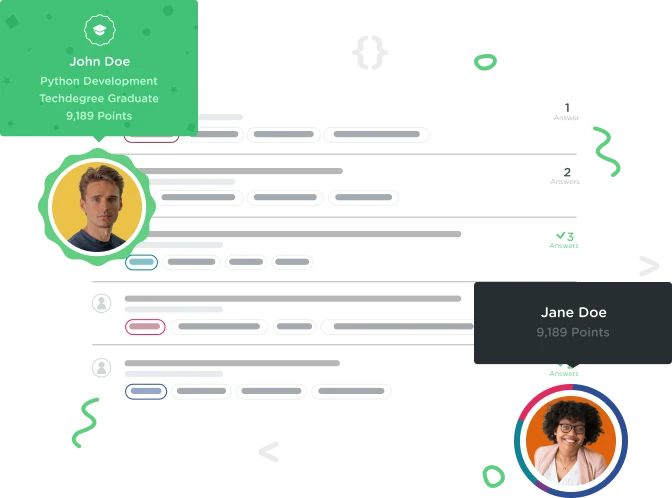
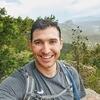
Justin Hein
14,811 PointsIntro to Prog - Function Extra Credit
Now, this time I got the code to work, but I can't help but feel that there is a better way to accomplish this.
var evenorodd = function (a, b, c) {
if (a%2 === 0) {
console.log("even");
} else {
console.log("odd")
}
}
evenorodd(4, 2, 4)
That's my code, and this was the challenge.
"Create a function that takes 3 arguments, a, b, and c. If a is an even number have the function return the string "even". Otherwise have the function return the string "odd""
Mine obviously will do, but I am wondering what other ways might work?
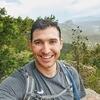
Justin Hein
14,811 PointsYes, just wondering if there is a better way/different way to do this. For instance, what would you do?
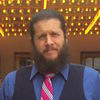
Adam Moore
21,956 PointsTo find odd or even? I would do the function the same, but with only one argument, since you aren't using b or c in that function. Well, I would probably write it like function evenorodd(a){ }
, instead of var evenorodd = function(a){ }
just because it says and does the same thing, but with less wording. `The reason you have to go through all of this trouble to find out if it is odd or even is because the terms "odd" and "even" are semantic, and only refer to whether or not a number is evenly divisible by 2. Therefore, that's what the function is checking: is the number divisible by 2.
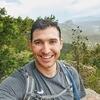
Justin Hein
14,811 PointsOkay awesome, so my actual method of checking for whether it is even or odd, using the if statements, is a good and valid way to do that?
4 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsIs there a better way? Not really.
But there's a shorter way, using the ternary operator:
function evenOrOdd(a, b, c) {
return a % 2 === 0 ? "even" : "odd";
}
It tests if a
is divisible by 2
. If it is, it returns "even"
, otherwise it returns "odd"
.
Now, if you could use a function that would return true
of false
, it could be even shorter (and simpler).
function isEven(a) { // I'm intentionally skipping b and c parameters here
return a % 2 === 0;
}
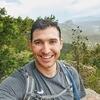
Justin Hein
14,811 PointsOkay, so I haven't learned about that yet, but that's really good to know! Why was Intro to Prog having me set the Function equal to a var if you just put the var before the arguments?

Dino Paškvan
Courses Plus Student 44,108 PointsThere are two ways of defining functions in JavaScript: function expressions and function statements.
A function statement (declaration) looks like this:
function myFunction() {
// some code
}
A function expression looks like this:
var myFunction = function() {
// some code
}
They behave slightly differently. When you cover hoisting in JavaScript Foundations you'll understand the difference a bit more, but the basic idea is that function statements are hoisted to the top during parse-time. Function expressions act more like normal variable assignments, so only the variable declaration is hoisted, not its definition (the actual function). A function expression is defined during run-time.
So basically, what this means is that you could call a function that was created using a function statement before that actual statement appears in the code.
A function defined with a function expression would return a TypeError, if called before its definition in the code.
Is one better than the other? Yes. Of course, which one is better depends on who you're asking. Myself, I used them interchangeably.
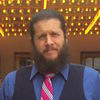
Adam Moore
21,956 PointsThanks, Dino, I hadn't heard of ternary before (not that I can remember), so I've learned a bit from this. I appreciate it, man!

Jason Anello
Courses Plus Student 94,610 PointsHi Justin,
Technically your function isn't correct because it doesn't return anything. It only logs to the console but you have the right general idea.
Based on the instructions it seems 'b' and 'c' do not factor into this at all.
One change you can make is to not use the else
block as it's not necessary.
var evenorodd = function (a, b, c) {
if (a%2 === 0) {
return "even";
}
return "odd";
}
evenorodd(4, 2, 4)
If the if
condition isn't true, you're just going to return "odd". Rather than having it fall through to the else
block you can simply let if fall through to the end of the function.
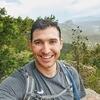
Justin Hein
14,811 PointsOh yeah, cause it ends at the first truthy statement right?

Dino Paškvan
Courses Plus Student 44,108 PointsA chain of if-else-ifs ends at the first truthy statement. Something else is at play here. If the condition is true, a return
statement is executed, it returns from the function, basically ending the execution of the rest of the function.
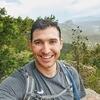
Justin Hein
14,811 Pointsokay, then visa versa, if it is false than the rest of the function will execute?

Dino Paškvan
Courses Plus Student 44,108 PointsIf a condition is false, yes, the code inside the if
statement gets skipped and the rest of the function is executed.
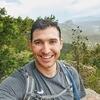
Justin Hein
14,811 PointsAwesome, thanks Dino!
Adam Moore
21,956 PointsAdam Moore
21,956 PointsAre you meaning another way to find out if a number is odd or even?