Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial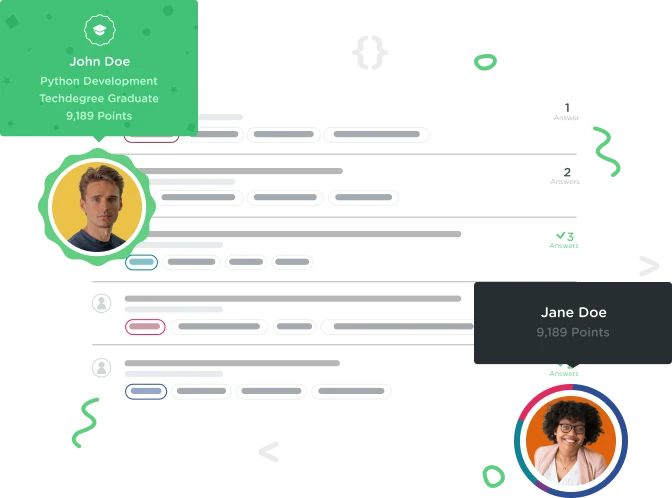
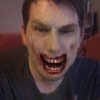
Mark Duplock
9,549 PointsIntro To Programming Deep Dive: Control Structures.
Hi all, I am having some trouble understanding why my JavaScript isn;t working for the Control Structures extra credit question:
"Write a program that loops through the numbers 1 through 100. Each number should be printed to the console, using console.log(). However, if the number is a multiple of 3, don't print the number, instead print the word "fizz". If the number is a multiple of 5, print "buzz" instead of the number. If it is a multiple of 3 and a multiple of 5, print "fizzbuzz" instead of the number."
My code:
var number = 100;
while (number > 0) {
if (number % 3 = 0) {
console.log("fizz");
}
if (number % 5 = 0) {
console.log("buzz");
}
else {
console.log(number);
}
number = number - 1;
}
This gives me the error: ReferenceError: invalid assignment left-hand side
I'm not looking for someone to just give me the answer, just a pointer or two would be awesome! Thanks!
15 Answers
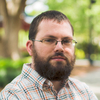
Kenneth Love
Treehouse Guest TeacherSo the equals sign (=
) has several jobs in JavaScript.
If it's all by itself between two items, like a = 2
, then it's doing what we call assignment, which is just a long word for saying that the label "a" now has the value of 2. We assigned 2 to a
.
If there's two of them, like a == 2
, then we're using it as an equality operator, which check to see if the two values are equivalent. So you're asking JavaScript if whatever value is in a
is equal to 2. This would be used, for example, inside of an if
statement.
There's also the case of using three equals signs, like a === 2
, which is kind of special to JavaScript. In our last example, a
could have had '2'
, the string version of 2, and it would still be equivalent because JavaScript can turn that string into a regular number. Using the threequals operator (as I like to call it), prevents JavaScript from changing the value to another type before doing the comparison.
If you look through your code, you'll see the places where you're doing assignment and should be doing equality checking.
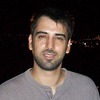
Michalis Efstathiou
11,180 Pointswell, I can see two issues there
first of all, = is an assignment operator, you cannot use it to check for equality
and second, the correct syntax for an if else statement in javascript that contains 2 or more conditions is
if (condition 1) {
} else if (condition 2) {
} else {
}
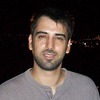
Michalis Efstathiou
11,180 Points@anello
Im confused now, if you use % 15, what happens when it gets to numbers lower than 15?
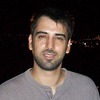
Michalis Efstathiou
11,180 Pointsoh ok thanks, i think i misunderstood what the question was asking, darn english

Roberto Alicata
Courses Plus Student 39,959 PointsYou used a single "=" to compare, but it means an assignment
if (number % 3 = 0 )
but you must use this:
if (number % 3 == 0 )
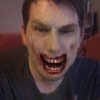
Mark Duplock
9,549 PointsAwesome guys, thanks!

Jason Anello
Courses Plus Student 94,610 PointsHi Mark,
Were you only interested in your syntax error? You have a logic error in your code as well and so you won't get the correct output.
Do you have that sorted out yet or need hints?
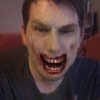
Mark Duplock
9,549 PointsHi Jason, I noticed this last night after fixing the syntax error. I will try and figure it out and if it gets the better of me I will be back :)
Thanks!
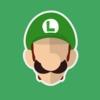
Bruno Dias
10,554 PointsHi guys,
While you're about it here is my code and my issue is that the console is not replacing the numbers with the words. What I did wrong?
for (var counter = 100; counter; counter = counter -1) {
console.log (counter);
if (counter % 3 == 0 && counter % 5 == 0) {
console.log ("fizzbuzz");
}
else if (counter % 3 == 0) {
console.log ("fizz");
}
else if (counter % 5 == 0) {
console.log ("buzz");
}
}

Jason Anello
Courses Plus Student 94,610 PointsSpoiler alert! Mark Duplock don't read Bruno's answer if you're still trying to figure this out on your own.
Hi Bruno,
You're pretty close here but you're logging the counter variable at the top of the loop which means you will log every single number in addition to one of those strings if appropriate. You only want to log the number if none of those conditions are true.
Hint: You need to add another block to your if/else if
structure that will get executed if none of the conditions are met. Log the number in there.

Jason Anello
Courses Plus Student 94,610 PointsI forgot one optimization you could make.
In your first if
condition you're checking if it's evenly divisible by both 3 and 5. A number evenly divisible by 3 and 5 is also evenly divisible by 15. So you could reduce the 2 modulo operations with a single one.
if (counter % 15 == 0) {
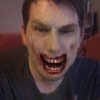
Mark Duplock
9,549 PointsHaha! Thanks Jason Anello - this is exactly how I solved it too.
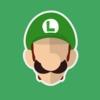
Bruno Dias
10,554 PointsThank you guys. The else at the end solved it.
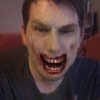
Mark Duplock
9,549 PointsMichalis Efstathiou There are no numbers below 15 that will evenly divide by both 3 and 5 so it will always be false.

Thiago de Bastos
14,556 PointsHey guys! Thanks for the help, here is my version! I structured it so that it would count up to 100 from 1.
for (var myNum = 1; myNum < 101; myNum = myNum + 1) {
if ((myNum % 3) + (myNum % 5) == 0) {
console.log("fizzbuzz");
} else if (myNum % 3 == 0) {
console.log("fizz");
} else if (myNum % 5 == 0) {
console.log("buzz");
} else {
console.log(myNum);
}
}
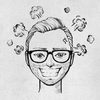
Ronny Gudvangen
4,685 PointsMy final version of this, after some syntax errors in the beginning (used to PHP) :p.
for (var number=1;number < 101;number = number + 1) {
if (number % 3 == 0 && number % 5 == 0) {
console.log("fizzbuzz");
} else if (number % 3 == 0) {
console.log("fizz");
} else if (number % 5 == 0) {
console.log("buzz");
} else {
console.log(number);
}
}
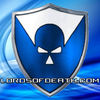
Ryan Grimsley
Courses Plus Student 1,386 PointsThis is how I did it. It looks like it works. I assume there are many ways to get this done, but this is the way that popped into my head at the time.
var counter = 1;
while(counter<101){
if(counter % 3 == 0 && counter % 5 == 0){
console.log("FizzBuzz")
counter=counter+1;
}
else if(counter % 3 ==0){
console.log("Fizz");
counter=counter+1;
}
else if(counter % 5 ==0){
console.log("Buzz");
counter=counter+1;
}
else{
console.log(counter);
counter=counter+1;
}
}