Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial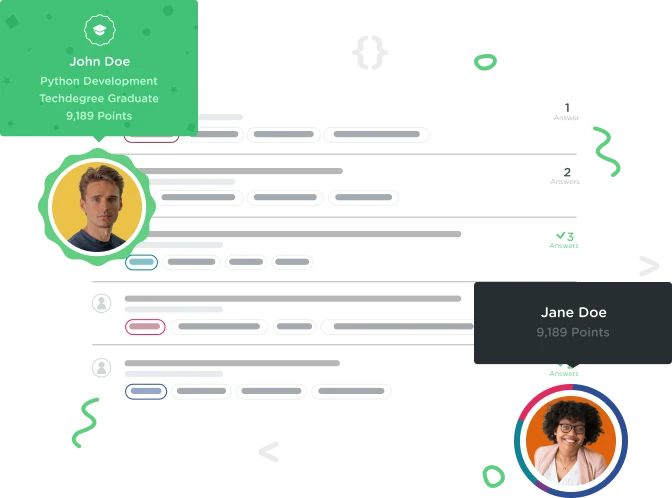

Thiago de Bastos
14,556 PointsIntro to Programming: Functions
Hey guys! I am trying to get the Extra credit done. What I want to add to this is the ability for the user to input the two values. It is late at night and I can't get my mind around it!
var evenOdd = function (b, c) {
var a = b + c;
if (a % 2 === 0) {
return "even";
} else {
return "odd";
}
}
console.log(evenOdd(1, 2));
This is what I have tried but can't get it to work:
var evenOdd = function (b, c) {
var a = b + c;
if (a % 2 === 0) {
return "even";
} else {
return "odd";
}
}
console.log(evenOdd(prompt("Enter a number"), prompt("Enter another number")));
For some reason I am getting odd or even based only on the last entered input. eg if I enter 1, 1 I get odd. If I enter 1, 2 I get even!
2 Answers
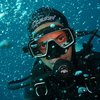
Nemanja Pribilovic
5,366 Pointsyou have to parseInt result from prompt parseInt(b) parseInt(c)

Thiago de Bastos
14,556 PointsThanks a lot guys!!
Nemanja Pribilovic
5,366 PointsNemanja Pribilovic
5,366 Pointsbecause you get string from prompt and you need to convert it to number
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherNemanja Pribilovic is right. If you type in 1 in the first prompt, then 2 in the second prompt, your JS code is going to do this
var a = "1" + "2"; // the result is 12, because this combines the 2 strings into one, bigger string.
You want to do this
var a = parseInt(a,10) + parseInt(b,10); // this will be 1 + 2 and will result in 3
The 10 in the
parseInt()
method is called a radix and makes sure the number is treated as a base ten, or decimal integer (instead of like an octal or hexadecimal value.