Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial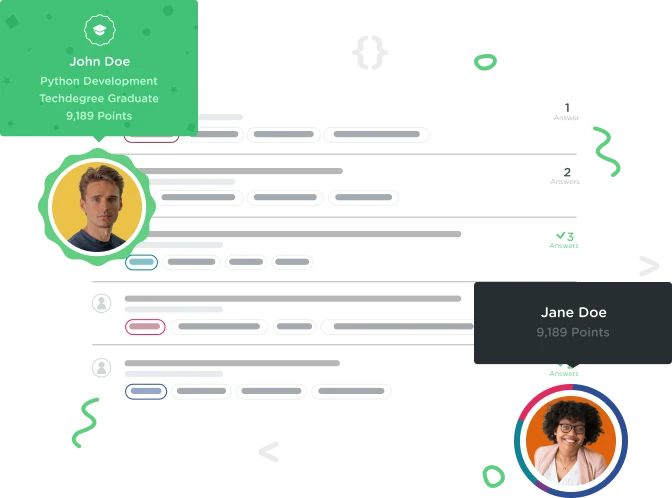

Willie Allison
2,035 Pointsinvalid argument supplied for foreach() is the code I am getting. How do I fix it?
I am having to put my code into my text editor because it is not working on the website code test area. I am getting a code invalid argument supplied for foreach?(). Any suggestions?
<?php
function mimic_array_sum($array) {
$total = 0;
foreach($array as $element) {
$total= $total + $element;
}
return $total;
}
$palindromic_primes = mimic_array_array(11, 757, 16361);
?>
2 Answers
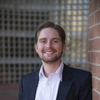
Stephen Van Delinder
21,457 PointsHey Willie,
Aaron is right, you have to be careful with typecasting in PHP, without explicitly saying that the input is an array, the computer won't really know what to do.
You are creating a function called mimic_array_sum() on line two. And calling a function named mimic_array_array() on line ten. Syntax is everything, and mimic_array_sum(array()); is very different than mimic_array_array();
<?php
function mimic_array_sum($array){
$total = 0;
foreach($array as $element) {
$total += $element;
}
return $total;
}
$palindromic_primes = array(11, 757, 16361);
// notice that you aren't echoing anything out or calling a function here,
// just creating an array that you will pass in later.
?>
Hope this helps.

Aaron Frey
6,672 PointsRight now you are passing three separate integer values to the function and not an array. You need to pass an array like this:
$palindromic_primes = mimic_array_array([11, 757, 16361]);
or
$palindromic_primes = mimic_array_array(array(11, 757, 16361));

Willie Allison
2,035 PointsThank you so much. With the help of you and Stephen I got to the next task but on it the code that worked in my text editor wasn't excepted by treehouse. Thank you again.
Willie Allison
2,035 PointsWillie Allison
2,035 PointsThank you so much.
Stephen Van Delinder
21,457 PointsStephen Van Delinder
21,457 PointsWillie,
No problem. If you utilize my code from above, the next challenge (if I remember correctly) should just be passing the array $palindromic_primes into the function mimic_array_sum(); and assigning it to a variable like this:
$sum = mimic_array_sum($palindromic_primes);
Then you should be able to echo the variable back out and move on.
Troubleshooting code on the treehouse platform can be difficult because (depending on the course) the interpreter may just say "Bummer, try again!" when there is a parse error. I use http://sandbox.onlinephpfunctions.com/ to figure out the line number and type of error.
PHP does not error out in a graceful way. The default error messages in PHP are cryptic, but it's good to be familiar with them. If the code fires in another editor, it's usually a format issue so some minor changes will get the code to pass on treehouse. Keep at it and it gets easier.
Hope this helps!