Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial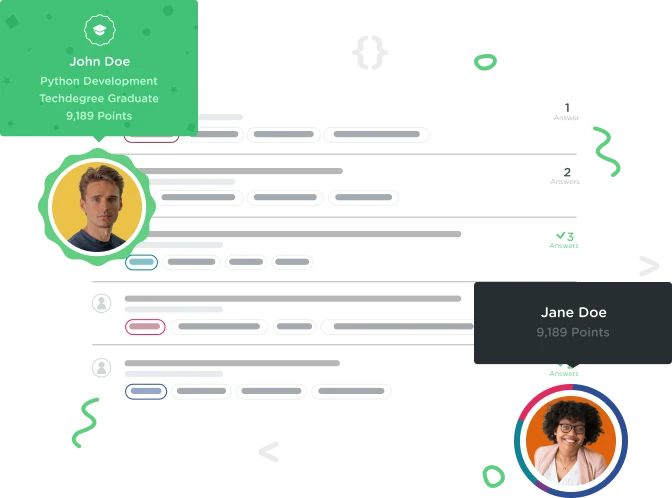

hyunsoo hwang
3,910 Pointsinvalid email
Basically, whatever I type in the username in the signing up page, pops an error message saying 'invalid email' but when I type in a username in form of an email, I am able to sign up. I can't seem to find what the problem is. I followed exactly as the tutorial video. Below is some codes where I think it is relevant.
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sign_up);
mUsername = (EditText)findViewById(R.id.usernameField);
mPassword = (EditText)findViewById(R.id.passwordField);
mEmail = (EditText)findViewById(R.id.emailField);
mSignUpButton = (Button)findViewById(R.id.signupButton);
mSignUpButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
String email = mEmail.getText().toString();
username = username.trim();
password = username.trim();
email = username.trim();
if (username.isEmpty() || password.isEmpty() || email.isEmpty()) {
AlertDialog.Builder builder = new AlertDialog.Builder(SignUpActivity.this);
builder.setMessage(R.string.signup_error_message)
.setTitle(R.string.signup_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
// create the new user!
ParseUser newUser = new ParseUser();
newUser.setUsername(username);
newUser.setPassword(password);
newUser.setEmail(email);
newUser.signUpInBackground(new SignUpCallback() {
@Override
public void done(ParseException e) {
if (e == null) {
// success!
Intent intent = new Intent(SignUpActivity.this, MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
else {
AlertDialog.Builder builder = new AlertDialog.Builder(SignUpActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.signup_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
});
}
<EditText
android:id="@+id/usernameField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:ems="10"
android:hint="@string/username_hint" >
<requestFocus />
</EditText>
Can someone please help me?
3 Answers

Ben Jakuben
Treehouse TeacherI had to run it in my own project and log the values to see the error. Notice anything wrong with these 3 lines?
username = username.trim();
password = username.trim();
email = username.trim();

Ben Jakuben
Treehouse TeacherIt almost sounds like your wires are crossed, in that somewhere the email and username fields are getting flipped or assigned incorrectly. Can you paste in your whole layout XML file? The username field looks okay, but perhaps the EditText for the email address accidentally has the same ID of "usernameField"?

hyunsoo hwang
3,910 PointsWow, I never thought I will get a reply from the lecturer himself. It really is an honor. I am really enjoying the course. Thank you so much !!!!!!!!!! I love your acting as well lol

Ben Jakuben
Treehouse Teacherlol thanks! We try to be as active as possible in here. Supporting you all is a big and fun part of our job!

hyunsoo hwang
3,910 Points<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".SignUpActivity" >
<EditText
android:id="@+id/usernameField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:ems="10"
android:hint="@string/username_hint" >
<requestFocus />
</EditText>
<EditText
android:id="@+id/passwordField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/usernameField"
android:layout_below="@+id/usernameField"
android:ems="10"
android:inputType="textPassword"
android:hint="@string/password_hint"
/>
<EditText
android:id="@+id/emailField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/passwordField"
android:layout_below="@+id/passwordField"
android:ems="10"
android:inputType="textEmailAddress"
android:hint="@string/email_hint" />
<Button
android:id="@+id/signupButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/emailField"
android:layout_below="@+id/emailField"
android:text="@string/sign_up_button_label" />
</RelativeLayout>
hyunsoo hwang
3,910 Pointshyunsoo hwang
3,910 PointsI see. So shouldn't it be
this? it makes sense now.
How silly of me. Sorry for not fully concentrating. I will focus harder and work harder. I promise Ben.
Thank you so much for personally helping me.
I am a big fan of yours.