Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial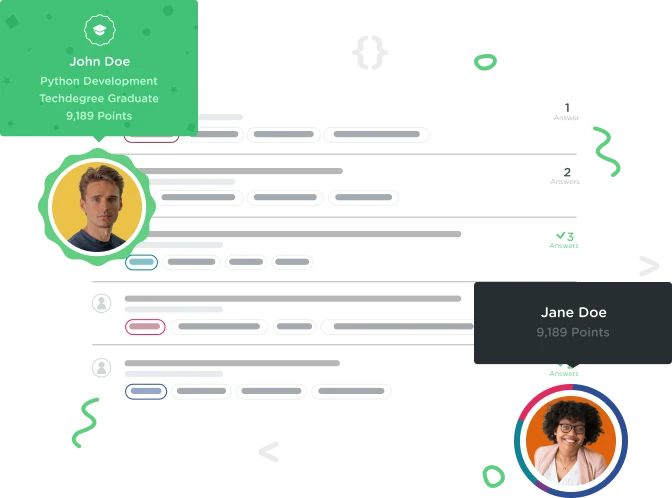

Naga Bala Krishnan A
905 Pointsinvalid literal for int() value?
TICKET_PRICE = 10
SERVICE_CHARGE = 2
tickets_remaining = 100
# Create the calculate_price function. It takes number of tickets and returns num_tickets * TICKET_PRICE
def calculate_price(number_of_tickets):
#creare a new constant for 2 dollar service charge
# Add the service charge to the result
return (number_of_tickets * TICKET_PRICE) + SERVICE_CHARGE
while tickets_remaining >= 1:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("What is your name: ")
num_tickets = input("Hello {}, How many tickets do you need?: ".format(name))
try:
num_tickets = int(num_tickets)
if num_tickets > tickets_remaining:
raise ValueError("There are only {} tickets remaining".format(tickets_remaining))
except ValueError as err:
print("Oh no. We ran into an issue. {}. Please try again.".format(err))
else:
ticket_price = calculate_price(num_tickets)
print("For {} tickets, the total price is {}".format(num_tickets, ticket_price))
prompt_answer = input("Do you want to purchase the ticket(s)? Y/N ")
if prompt_answer.lower() == "y":
print("Purchase of {} tickets confirmed. SOLD!".format(num_tickets))
tickets_remaining -= num_tickets
else:
print("Thanks for showing interest in us, {}".format(name))
print("Sorry the tickets are all sold out! :(")
When I give a string value to the question "How many tickets you need?" , i get "Oh no. We ran into an issue. invalid literal for int() with base 10: 'n'. Please try again."
Why am i getting invalid literal for int() error?
Mod Edit: Added code markdown to make code easier to read.
3 Answers
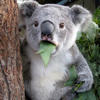
ursaminor
11,271 PointsBecause you can't turn a string into an int (unless the string is of a number). On this line:
num_tickets = int(num_tickets)
If the input was a number, you could turn it into an int and it would continue. If it was not a number, it would raise ValueError and the code under the except clause would run. That's why you got the message.
except ValueError as err:
print("Oh no. We ran into an issue. {}. Please try again.".format(err))

andren
28,558 PointsThe int
function takes a string that contains a literal number, like say "8"
and converts it into an int
of the same number 8
, which is needed in order to perform math operations on it. It is not designed to deal with strings that contains letters or words.
So the reason why passing in "n" results in that message is that "n" is indeed not a valid input for int
to process. This is also partly why the code is wrapped in a try
block to begin with. To catch instances of people typing in something besides a number and giving them a chance to try again, instead of having the program instantly crash. So the behavior you are witnessing is not a bug, but the program working exactly as intended.

Naga Bala Krishnan A
905 PointsThanks, Andren. So in this case how will I be able to give that error message in a more user friendly way?

Vincent Cegers
1,248 PointsThis being said shouldn't we have a second error telling the user what is wrong?

jeff Jackson
1,169 PointsI had a similar thought. It may not be pretty, but I handled it by using the find method and an if statement to search for the keyword remaining (thus differentiating the two types of error codes we tried to handle). see section of code below:
except ValueError as err: err_type = str(err) if (err_type.find("remaining") != -1): print("Enter a valid ticket value. {}. Please try again.".format(err)) else: print("Enter a valid ticket value. Please try again.")
Naga Bala Krishnan A
905 PointsNaga Bala Krishnan A
905 PointsThanks ursaminor. Appreciate your answer. I now understand it. Cheers!