Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial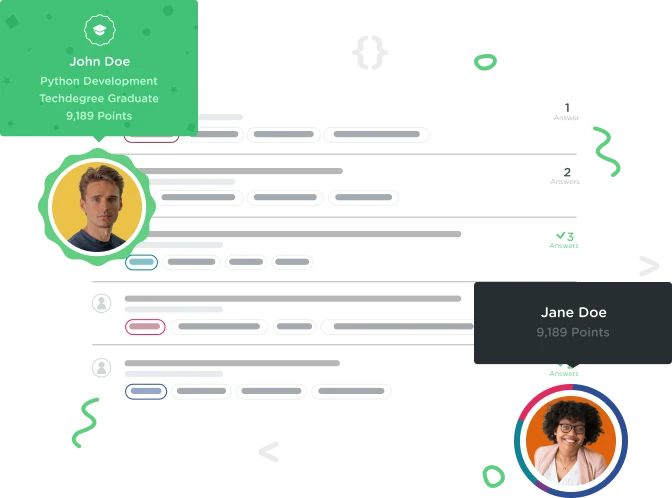
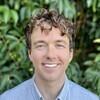
Asher Orr
Python Development Techdegree Graduate 9,410 PointsInvalid Literal for Int() with Base 10: How to raise a ValueError when users enter a non-integer for num_tickets.
Hi everyone! When I completed this project, I noticed that the console would deliver a funky message when I entered a non-integer (like "blue") when answering how many tickets I'd like to purchase.
The error said: invalid literal for int() with base 10: ''
Here's the code I'm working with:
try:
num_tickets = int(input("How many tickets would you like to buy? "))
if num_tickets > tickets_remaining:
raise ValueError(("There are only {} tickets left").format(tickets_remaining))
elif num_tickets != int():
raise ValueError("Please enter only a number when purchasing tickets")
except ValueError as err:
print("Oh no! We ran into an issue. {}. Please try again.".format(err))
From my understanding, this code will raise the first ValueError when num_tickets > tickets_remaining. But if that's not true, the code will then check to see if num_tickets is not equal to an integer.
If that is true, then the code should raise the second ValueError "Please enter only a number when purchasing ticket.)
I've tried several iterations, but I can't seem to get my code to refer to the ValueError "Please enter only a number when purchasing tickets" when I enter a non-integer.
Can anyone help me here? What am I missing?
1 Answer

jb30
44,807 Pointsnum_tickets = int(input("How many tickets would you like to buy? "))
gets input from the user, then tries to convert the input to an integer. If the conversion fails, it throws a ValueError
. If you want to have a specific error message if it isn't an integer, you could place another try-except
block around it, such as
try:
try:
num_tickets = int(input("How many tickets would you like to buy? "))
except ValueError:
raise ValueError("Please enter only a number when purchasing tickets")
else:
if num_tickets > tickets_remaining:
# ...
elif num_tickets != int():
compares num_tickets
to the result of int()
. Since no argument is passed to the int
function, int()
will return 0
. If num_tickets
is not 0
, the elif
statement is true, so the next line will raise the ValueError
.
Asher Orr
Python Development Techdegree Graduate 9,410 PointsAsher Orr
Python Development Techdegree Graduate 9,410 PointsHey JB, thanks for your help! It worked! I really appreciate you!
Would you mind explaining why you need to nest that within an additional try block, though? I'm not understanding why that's necessary.
jb30
44,807 Pointsjb30
44,807 PointsWithout the additional
try
block, invalid input fornum_tickets = int(input("How many tickets would you like to buy? "))
will be thrown toand
err
will be something likeinvalid literal for int() with base 10: 'blue'
and not the custom error message"Please enter only a number when purchasing tickets"
.To avoid using a nested
try-except
block, you could change theexcept
block towhich will change
err
to"Please enter only a number when purchasing tickets"
when the error message contains the string"int()"
.