Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial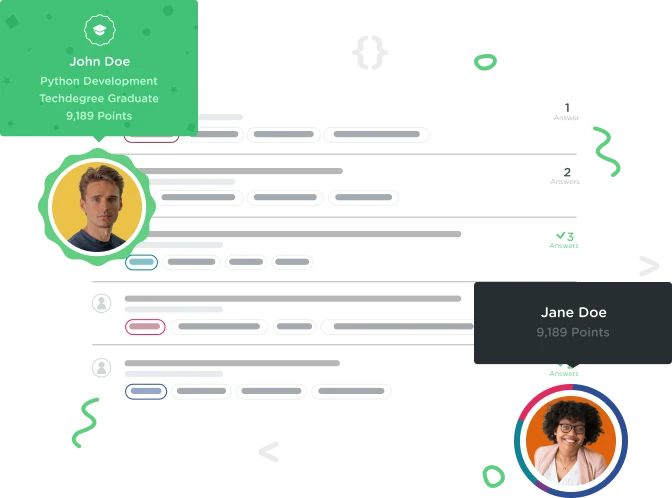

Ruben Arellano
732 Points"Invalid literal" when entering a character into a int question
Whenever I put a character into the "how many tickets" prompt, I get this "invalid literal". Any idea what am I doing wrong?
Script:
try: tix_purchase = int(input("Hello {}.\n How many tickets would you like? ".format(name))) if ( tix_purchase > tickets_remaining ) : raise ValueError (" We don't have {}.".format(tickets_remaining)) except ValueError as err: print ("Uh oh! Invalid entry . {} Please try again.".format(err))
Console:
Please enter your name ruben
Hello ruben.
How many tickets would you like? jk
Uh oh! Invalid entry . invalid literal for int() with base 10: 'jk' Please try again.
There are 100 tickets available
1 Answer
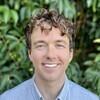
Asher Orr
Python Development Techdegree Graduate 9,410 PointsHi Ruben! You are seeing that error because of this line of code:
tix_purchase = int(input("Hello {}.\n How many tickets would you like? ".format(name)))
Specifically this:
int
That says "Python, what my user inputs/enters is going to be an integer! Throw an error if they enter anything that isn't an integer."
Since "jk" is a string, and not an integer, Python raised that ValueError.
Does that make sense?
PS- check out the markdown cheatsheet for syntax examples on how to format your post. It will make your code easier to read!
Tricia Cruz
915 PointsTricia Cruz
915 PointsHi Asher Orr , I came across a similar problem with the code below:
when I try to to give an input of "hi" (for example) when prompted "How many tickets...", I get
It looks like we've come across an error. invalid literal for int() with base 10: 'hi' Please try again.
I originally had my code as
And it came with the same issue just laid out differently so I watched the video... then we're never shown another run where a string's inputted in that prompt.
Is it really meant to come out that way? I looked back on the video and they did the same with the "tickets" variable...
edit: Oh. Okay, I think I get it. Is it because I need to do another except block, but this time for a TypeError?
edit 2: Okay that seemed to work when I did this:
My question is though, is there a more concise way of doing this 😬 Unless I'm getting ahead of myself and this is going to be covered in the next video
Asher Orr
Python Development Techdegree Graduate 9,410 PointsAsher Orr
Python Development Techdegree Graduate 9,410 PointsHi Tricia Cruz, great question.
You should check out this post on StackOverflow.
I put the code above into my IDE, and I got a TypeError no matter what I entered. It's because of these lines:
The second line (in the code above) re-defines the tickets variable to be... well, whatever it was before! Since "tickets" is defined by the user's input, it will be a string (user input is always a string.)
When the script gets to the next line (if tickets > tickets_remaining), it throws this error:
TypeError: '>' not supported between instances of 'str' and 'int'
That's because tickets_remaining is an integer, but tickets is a string.
You could make your code more efficient like this:
Remember:
When we convert the user_selection input to an integer, Python will check to see if it's the right type. It will check for a string, since user input is always a string. Then it checks for an appropriate value- a string that can be converted into an integer (like "8").
Python will consider any non-number string as an inappropriate value. Thus, it will throw a ValueError. You can simplify the error handling that way.
Does this answer your question?
Tricia Cruz
915 PointsTricia Cruz
915 PointsYes, thank you so much!!