Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial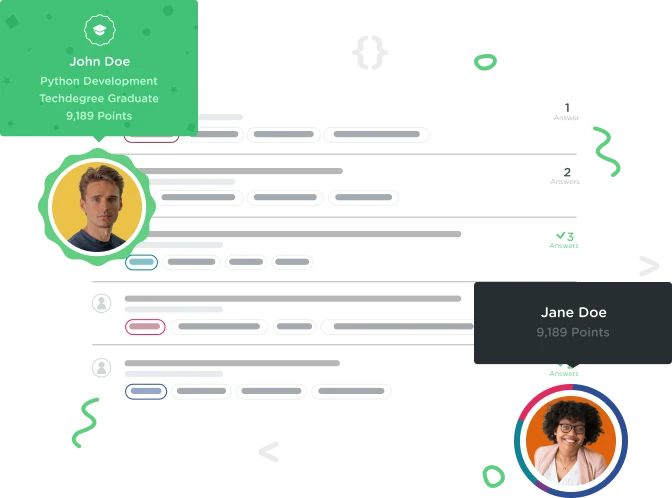
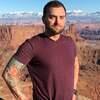
Mitchell Wintrow
4,380 PointsInvalid status code. What is that and why doesn't it work?
Hi guys! Thanks for all your help. I'm posting this on here because I read many posts, but unfortunately everything I've tried still hasn't fixed my problem and I don't understand why. I've followed along exactly as Andrew did in the video, but to be honest he gets kind of confusing sometimes by jumping around a lot or changing stuff with the expectation that we should just know what should change back or delete. I keep getting this error:
RangeError [ERR_HTTP_INVALID_STATUS_CODE]: Invalid status code: undefined
at ServerResponse.writeHead (_http_server.js:259:11)
at ServerResponse._implicitHeader (_http_server.js:250:8)
at write_ (_http_outgoing.js:711:9)
at ServerResponse.end (_http_outgoing.js:825:5)
at ServerResponse.send (/Users/mitchwintrow/Desktop/Treehouse/Flashcards/node_modules/express/lib/response.js:221:10)
at done (/Users/mitchwintrow/Desktop/Treehouse/Flashcards/node_modules/express/lib/response.js:1008:10)
at Object.exports.renderFile (/Users/mitchwintrow/Desktop/Treehouse/Flashcards/node_modules/pug/lib/index.js:448:12)
at View.exports.__express [as engine] (/Users/mitchwintrow/Desktop/Treehouse/Flashcards/node_modules/pug/lib/index.js:493:11)
at View.render (/Users/mitchwintrow/Desktop/Treehouse/Flashcards/node_modules/express/lib/view.js:135:8)
at tryRender (/Users/mitchwintrow/Desktop/Treehouse/Flashcards/node_modules/express/lib/application.js:640:10)
Here is my app.js:
const express = require('express');
const bodyParser = require('body-parser');
const cookieParser = require('cookie-parser');
const app = express();
app.use(bodyParser.urlencoded({extended: false}));
app.use(cookieParser());
app.set('view engine', 'pug');
const mainRoutes = require('./routes');
const cardRoutes = require('./routes/cards');
app.use(mainRoutes);
app.use('/cards', cardRoutes);
app.use((req, res, next) => {
const err = new Error('Not found!');
err.status = 404;
next(err);
});
app.use((err, req, res, next) => {
res.locals.error = err;
res.status(err.status);
res.render('error');
});
app.listen(3000, () => {
console.log('The application is running on localhost:3000!')
});
Here's my index.js:
const express = require('express');
const router = express.Router();
router.get('/', (req, res) => {
const name = req.cookies.username;
if (name) {
res.render('index', {name});
} else {
res.redirect('/hello');
}
});
router.get('/hello', (req, res) => {
const name = req.cookies.username;
if (name) {
res.redirect('/');
} else {
res.render('hello');
}
});
router.post('/hello', (req, res) => {
res.cookie('username', req.body.username);
res.redirect('/');
});
router.post('/goodbye', (req, res) => {
res.clearCookie('username');
res.redirect('/hello');
});
module.exports = router;
Here's my cards.js:
const express = require('express');
const router = express.Router();
router.get('/cards', (req, res) => {
res.render('card', {prompt: "Who is buried in Grant's tomb?"});
});
module.exports = router;
And finally here's my card.pug just to be safe:
extends layout.pug
block content
section#content
h2= prompt
if hint
p
i Hint: #{hint}
1 Answer

jb30
44,807 PointsIn app.js
, you have app.use('/cards', cardRoutes);
. In cards.js
, you have a method starting with router.get('/cards', (req, res) => {
. The routes are combined, so you would find the cards
page at localhost:3000/cards/cards
. If instead you wanted the cards
page to be at localhost:3000/cards
, you could change either of the '/cards'
to '/'
, but not both.
For the original error, in app.js
, you had
app.use((err, req, res, next) => {
res.locals.error = err;
res.status(err.status);
res.render('error');
});
This assumes that err.status
has been set to a particular value, such as 404
. If err.status
has not been set to a value, its value is undefined
, which makes res.status(err.status)
equivalent to res.status(undefined)
, which sets the status code to undefined. You could try setting the status code to err.status
only if err.status
has a truthy value by
if (err.status) {
res.status(err.status);
}
You could then look at the error page in your browser to see why you had an error.
Mitchell Wintrow
4,380 PointsMitchell Wintrow
4,380 PointsJust an update for whoever is responds to my question, I found out how to get past that error but instead discovered another error that prevents me from moving on from the video that follows this video. I had to change the
app.use('/cards', cardRoutes);
toapp.use(cardRoutes);
. Now my new problem is this:When I have my cards.js file set up like this:
it works and I can view the cards page finally. But in the video Andrew said we can just change
router.get('/cards', (req, res) => {
torouter.get('/', (req, res) => {
and it will still work. When I change mine to just have'/'
it stops working and I get a 404 error so I left it as'/cards'
and then it works again. So I followed along in the next video but as soon as I tried doing what he said:'/cards:id'
andprompt: cards[req.params.id].question, hint: cards[req.params.id].hint
then it stopped working and I got a 404 error again when I typed this into the url:localhost:3000/cards/0
.Can anyone please help me to understand what is going on? Andrews videos are really confusing. I wish Guil was teaching this stuff because I'm really interested in Node and Express and all the server side stuff, and Guil is meticulous and doesn't skip over anything or assume we know something. He just gives it all to us, simple, concise, and meticulous with the details.