Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial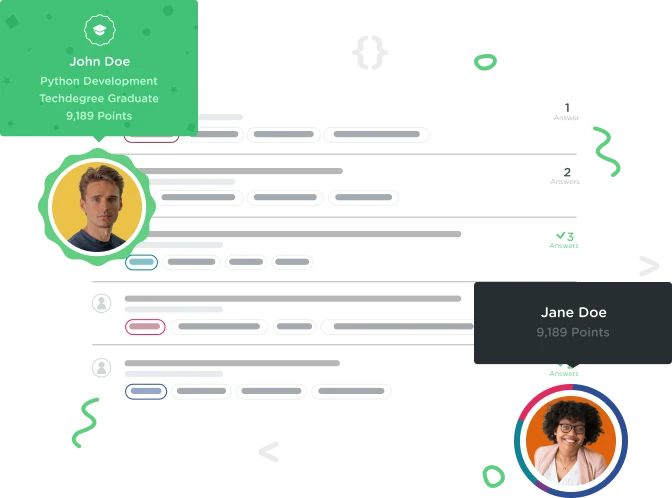

Jorge Grajeda
Python Development Techdegree Graduate 8,186 PointsInventory.py
Could somebody please help me out for the last part of this challenge?
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def add_item(self,item):
super()add_item(item)
def list.sort():
self.sort()
2 Answers

jb30
44,806 PointsInstead of super()add_item(item)
, you could do super().add_item(item)
.
You don't need to define list.sort()
. It's a built in method. You can pass the method your list as an argument, such as list.sort(your_list)
, and your list will be sorted.

Jorge Grajeda
Python Development Techdegree Graduate 8,186 PointsCould you explain it a little bit more? I'm still a little confused lol

jb30
44,806 PointsTo call a method from within a class, you usually use the form self.add_item(item)
. When you want to run the superclass's implementation of the method, you can replace self
with super()
. You will still need to include the .
to call the method.
If you have a list such as
some_list = [2, 1, 3]
print(some_list) # prints [2, 1, 3]
some_list.sort()
print(some_list) # prints [1, 2, 3]
Calling sort()
directly on the list some_list
is equivalent to using list.sort(some_list)
, and the list is sorted in place, meaning that sort()
modifies whatever list you use with it. It's a built-in method of lists, meaning that all lists in python are able to be sorted in this way without needing additional code.
The class Inventory
has the list slots
, which you can access from within its subclass SortedInventory
as self.slots
. To sort the list self.slots
, you can use self.slots.sort()
or list.sort(self.slots)
.