Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial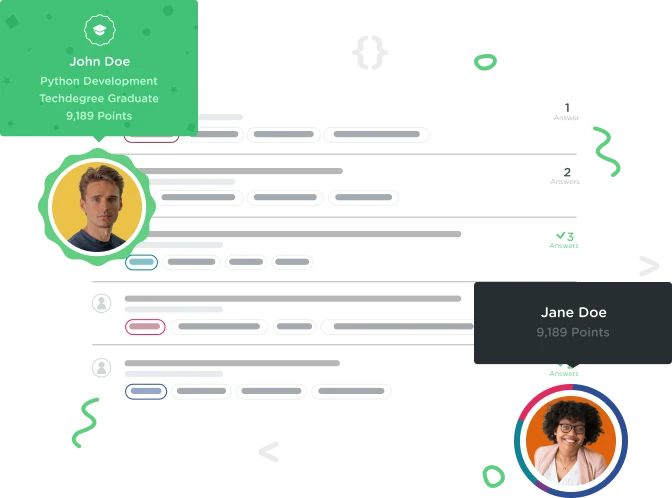
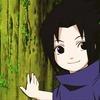
Matthew Francis
6,967 PointsInvoking a function and "this" keyword
var Button = function(content) {
this.content = content;
};
Button.prototype.click = function() {
console.log(this.content + ' clicked');
}
//First way of invoking
var myButton = new Button('OK');
myButton.click();
//Second Way of invoking
var looseClick = myButton.click;
looseClick();
//What is the purpose of invoking a function like this? is there for a name for this way of invoking?
//How is the first way of invoking different than this way?
// Why is "this" the window object here?
1 Answer
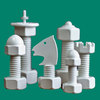
Steven Parker
231,275 PointsThe value of "this" depends on the context.
When invoking an object method (like your first example), "this" is the object itself. But when invoking a function in global code (like your second example), it is the window object.
If you wanted to "looseClick" to not be "loose", you could bind it when you assign it:
var looseClick = myButton.click.bind(myButton);
You might enjoy the short workshop on Understanding "this" in JavaScript.