Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial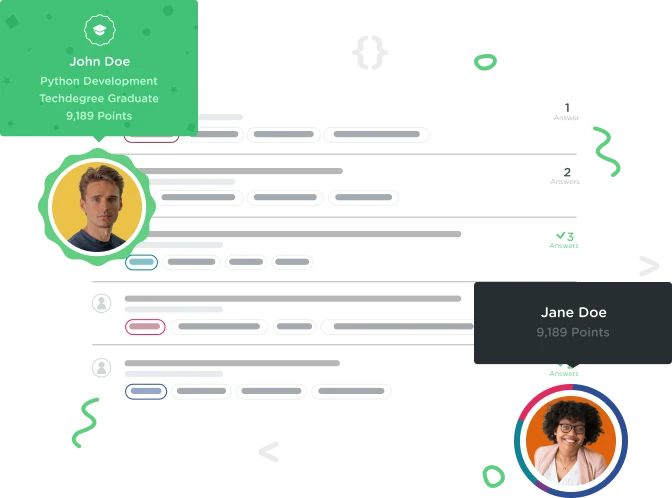
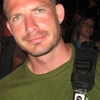
Drew Wolfe
3,140 PointsiOS | Creating a Custom Class | Challenge 2 of 3
Switch to the implementation file and implement a 'quotes' method which will allocate and initialize the _quotes instance variable only if it is 'nil'. Initialize the array with any three strings.
#import "Quote.h"
@implementation Quote
- (NSArray *) quotes {
if (_quotes == nil) {
_quotes = [[NSArray alloc] initWithObjects:@"one", @"two", @"three", nil];
}
return _quotes;
}
- (NSString *) randomQuote {
return @"Some Quote";
}
@end
Bummer! Remember to allocate and initialize the instance variable '_quotes' and return it from the method named 'quotes'.
I just don't know what I'm doing wrong. I had the same response as above, but with a different set of strings... fail. Then I literally copied a highly rated forum response (https://teamtreehouse.com/forum/code-challenge-creating-a-custom-class-2#) in order to ensure getting it right... fail. I've actually had the correct response a couple of times (on other challenges) and simply had to open the challenge (again) in another tab (OS X 10.9.2, Chrome Version 33.0.1750.152), but this doesn't seem to be working either.
2 Answers
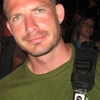
Drew Wolfe
3,140 PointsThanks Patrick!
It ended up that I got ahead of myself and used the "readonly" attribute in the property creation from the previous task. The first challenge accepted it (as I guess it wasn't technically "wrong"), but it got in the way of the second challenge. Here's what I submitted and passed with in the previous challenge:
@interface Quote : NSObject
// Add property here
@property (strong, nonatomic, readonly) NSArray *quotes;
- (NSString *) randomQuote;
@end
I wish I could say I figured it out on my own, but Amit figured it out and posted in a related post (https://teamtreehouse.com/forum/code-challenge-creating-a-custom-class).
But seriously, thanks for the speedy response man. This is an awesome learning platform with an awesome support response.
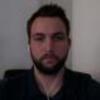
Patrick Donahue
9,523 PointsTry self.quotes = [[NSArray alloc] initWithObjects:@"one", @"two", @"three", nil];
Patrick Donahue
9,523 PointsPatrick Donahue
9,523 PointsNo problem! Glad you found your answer!