Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial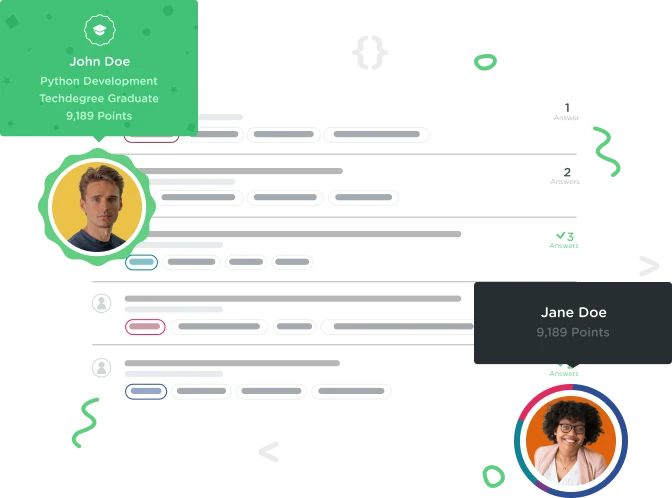

Chris Sommers
365 PointsiOS Email Validation with modal alert in Swift
I am a beginner with Swift, iOS, and Xcode. I am working on a multi-scene grading app done through a storyboard in Xcode 7 for a class assignment. One of my scenes is a register page and I need to validate the email.
Here's what I have, the following code is on the view controller that pertains to the register page and outlets exist for each field. I am trying to use shouldperformseguewithidentifier to either allow or prevent the segue on the register button from taking place - not working. However, I know my email validation method is working because I can manually test it in a playground and see it. When I run the app, the segue goes right to the menu regardless of the validity of the email text. I'm not too sure if the actual value is coming from the textfield. I'd like to use a print statement to check but I don't know where the output is shown while testing in the simulator.
So the desired outcome of the code below which is generating no errors or warnings is to stop the segue if the email is invalid and then to present a modal alert to the user using UIAlertController. Can someone please tell me what I'm doing wrong?
class StudentRegisterKappa: UIViewController {
@IBOutlet weak var firstName: UITextField!
@IBOutlet weak var lastName: UITextField!
@IBOutlet weak var email: UITextField!
@IBOutlet weak var phoneNumber: UITextField!
@IBOutlet weak var userName: UITextField!
@IBOutlet weak var password: UITextField!
override func shouldPerformSegueWithIdentifier(identifier: String, sender: AnyObject?) -> Bool {
if identifier == "kappaToMenu" {
if validateEmail(email.text!) {
return true
} else {
let alertView = UIAlertController(title: "Invalid Email", message: "Try Again", preferredStyle: .Alert)
alertView.addAction(UIAlertAction(title: "Ok", style: .Default, handler: nil))
presentViewController(alertView, animated:true, completion:nil)
return false
}
}
//default
return true
}
func validateEmail(candidate: String) -> Bool {
let emailRegex = "[A-Z0-9a-z._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,6}"
return NSPredicate(format: "SELF MATCHES %@", emailRegex).evaluateWithObject(candidate)
}
}
2 Answers
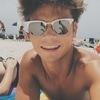
garyguermantokman
21,097 PointsYou can just use performSegueWithIdentifier if the email is valid, segue to the new view controller.
if validateEmail(email.text) {
// Success
performSegueWithIdentifier("SEGUE-ID", sender: self)
} else {
// Failure - Alert
}
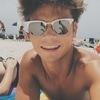
garyguermantokman
21,097 PointsYou can use one of the UITextFieldDelegate methods to get the text of the textField. Then make the above check. Check out the docs on UITextFieldDelegate.
Chris Sommers
365 PointsChris Sommers
365 PointsWhere do I do that? If I just place that conditional in the body of the class I get a declaration error. The segue was created on the storyboard by cntrl dragging from a button to another view so I am assuming I need to override normal segue behavior and then perform a conditional to either allow the segue to continue or stop it. Clearly though, I am not doing this right.