Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial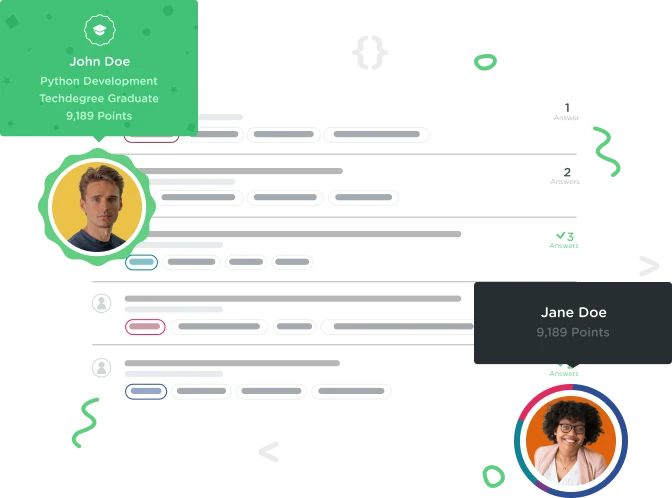

Rushabha Jain
Full Stack JavaScript Techdegree Student 11,140 PointsIs constructor method compulsory?
Is constructor method inside the class compulsory even if you don't won't to initialize with starting parameters?
2 Answers

Sam oomen
1,063 PointsIt is not compulsory to define a constructor method for a class in JavaScript. If a class does not have a constructor method, JavaScript will automatically create a default constructor for the class when an object is created from the class. The default constructor simply creates and initializes the object, and does not have any arguments or additional functionality.
Here is an example of a class with a default constructor:
class MyClass {
// No constructor method defined
}
const obj = new MyClass(); // A default constructor is automatically created
You can also define your own custom constructor method for a class in JavaScript, which allows you to specify the arguments and functionality of the constructor. Here is an example of a class with a custom constructor method:
class MyClass {
constructor(arg1, arg2) {
this.arg1 = arg1;
this.arg2 = arg2;
}
}
const obj = new MyClass(1, 2); // The custom constructor is called with the arguments 1 and 2
So, it is not compulsory to define a constructor method for a class in JavaScript, but you can define a custom constructor if you want to specify the arguments and functionality of the constructor.
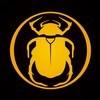
rydavim
18,814 PointsTake a look at the MDN constructor page for all the ins and outs of this. The short answer is if you don't define a constructor method, your object will use a default one.
If you do not specify a constructor method, a default constructor is used.
Default Constructors:
// base classes
constructor() {}
// derived classes
constructor(...args) {
super(...args);
}
Hopefully that helps, but let me know if you have any questions. Happy coding!