Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial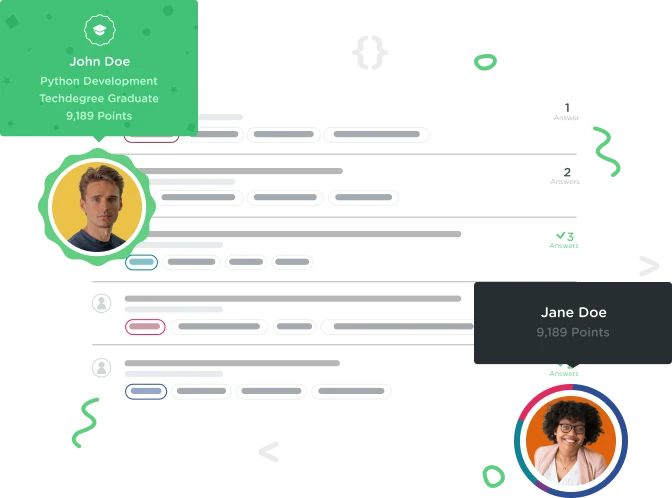

Erik Embervine
Python Development Techdegree Student 2,442 Pointsis Craig's code in the masterticket.py program considered "clean" or "complete"?
after Craig completes his finishing touches to the code in this video, i ran the code again. when i enter a string value to answer the question "How many tickets would you like?", it outputs not only the "Sorry not valid" print statement in the "except ValueError as err" block, which makes sense, but it also prints out the contents of the "err" variable: "invalid literal for int() with base 10: [string]". is this what Craig intended? i assume we don't want users to see this.. maybe i missed something? i came up with my own solution to handle the message, but was curious if i am mistaken, or if this was an oversight or by design? other?
1 Answer
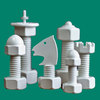
Steven Parker
229,744 PointsI think you can expect that every course exercise will be designed to illustrate the lesson principles but none will be examples of fully implemented and rigidly tested ready-to-publish software products.
The fact that all the examples may be efficiency optimized, UX enhanced, or given additional features can become opportunities for extra practice if you want some.
As to improvements, this line of the code caught my eye:
if purchaseConfirmation.startswith('y' or 'Y'):
You can't combine terms with logic operators, only complete expressions. This won't detect a capital "Y". But you can do it with complete expressions like this:
if purchaseConfirmation.startswith('y') or purchaseConfirmation.startswith('Y')::
But perhaps more efficiently like this:
if purchaseConfirmation.lower().startswith('y'):
Also, testing for ticketCount above 100 or less than 0 isn't necessary, since neither is a possible condition.

Erik Embervine
Python Development Techdegree Student 2,442 Pointsactually it seems i can enter -100 for the ticket count and is accepted, because ticketCount isn't handled anywhere for values less than 0 and it's different than the tickets remaining variable...
so i added this:
TICKET_PRICE = 10
SERVICE_CHARGE = 2
tickets_remaining = 100
def calculateTotal(ticketCount):
return ticketCount * TICKET_PRICE + SERVICE_CHARGE
def masterticket(TICKET_PRICE,tickets_remaining):
print("There is/are ",tickets_remaining," tickets left!")
newVisitor = input("What is your name? ")
while True:
try:
ticketCount = int(input("How many tickets would you like, "+newVisitor+"?"))
#handle numbers higher than remaining tickets. we've already handled tickets < 0 in a previous while loop
if ticketCount > tickets_remaining or ticketCount <= 0:
print("Sorry we don't have that many..")
continue
break
#handle string value exceptions
except ValueError:
ticketCount = print("Sorry, I need you to give me a number...")
continue
totalPrice = calculateTotal(ticketCount)
print("Ok, your total comes to: $",totalPrice)
purchaseConfirmation = input("Do you want to proceed with purchase? Y/N: ")
if purchaseConfirmation.lower() == 'y':
tickets_remaining -= ticketCount
print("Thanks for your purchase!")
else:
print("No problem, thanks for visiting!")
return tickets_remaining
tickets_left = masterticket(TICKET_PRICE,tickets_remaining)
while tickets_left > 0:
tickets_left = masterticket(TICKET_PRICE,tickets_left)
print("Monty Python Show Tickets are SOLD OUT!!!")
....i think it's solid now...
Erik Embervine
Python Development Techdegree Student 2,442 PointsErik Embervine
Python Development Techdegree Student 2,442 PointsFYI, this is how I solved it myself, but am wondering if there is a better/more efficient way...