Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial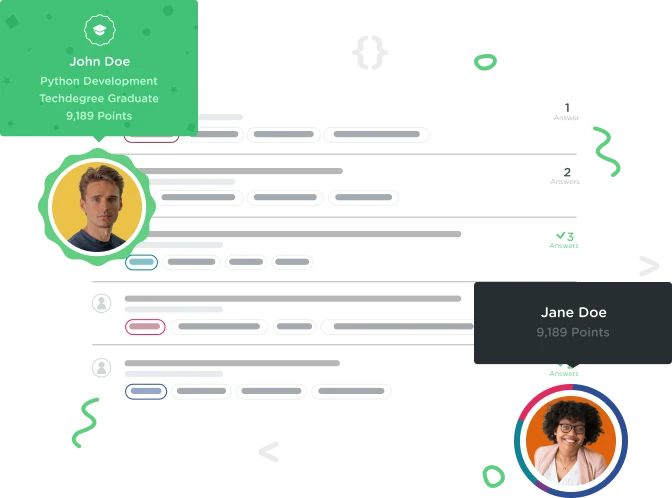

Damar Wicaksono
5,562 PointsIs __iadd__ method supposed to change the data type of NumString?
Following the example in the video, adding an integer in place to an object of NumString class seems to change its data type.
Is this an expected behavior (perhaps it stems from the fact that adding the object with a number returns either float or int thus overwrite the original object)? Should we simply return self
instead of self.value
in the __iadd__
method? Or did I miss something?
2 Answers

Jon Boettcher
7,343 PointsI had the same question on this and I believe that is what's happening. The code given in the video is:
class NumString:
def __init__(self, value):
self.value = str(value)
def __str__(self):
return self.value
def __int__(self):
return int(self.value)
def __float__(self):
return float(self.value)
def __add__(self, other):
if '.' in self.value:
return float(self) + other
else:
return int(self) + other
def __radd__(self, other):
return self + other
def __iadd__(self, other):
self.value = self + other
return self.value
Then the example usage is:
>>> age = NumString(25)
>>> age += 1
>>> age
26
If age was still a NumString object, typing only ">>> age" into the console should have output something like "<numstring.NumString object at 0x7f4e251826d8>"
Looking at the Python documentation on iadd, using age += 1 is equivalent to age = age.iadd(1), so the return value of iadd is being assigned back to age, in this case the integer 26.
I tried a different implementation by returning self, rather than self.value. Additionally, I also converted the result of the add operation to a str, since the NumString object is expecting a string for its value attribute.
def __iadd__(self, other):
self.value = str(self + other)
return self
This seems to do what's expected:
>>> age = NumString(25)
>>> age += 1
>>> age
<numstring.NumString object at 0x7f5fe6cdb668>
>>> print(age)
26

YURI PAPINIAN
11,838 PointsGreat point, Jon. I face the same problem. Yes, Jon's solution works for iadd. However, it doesn't work for imul. How come?