Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial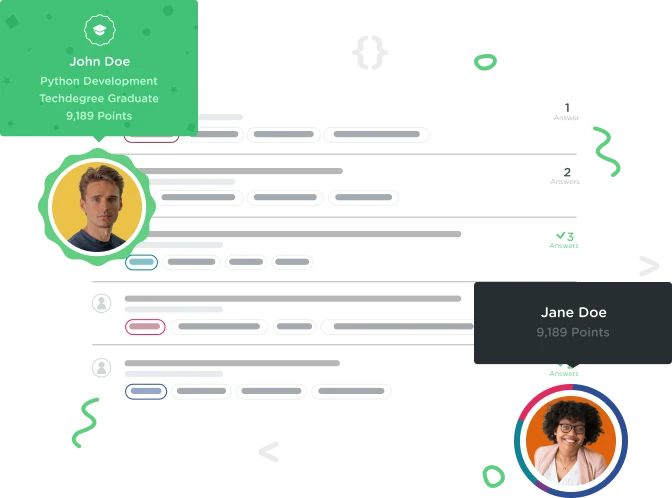

Diego Alvarez
6,680 PointsIs it a good practice if I do it like this?
alert('Let\'s do some math!');
var input1 = parseFloat(prompt('Please type a number'));
var input2 = parseFloat(prompt('Please type another number'));
var sumNums = input1 + input2;
var multiplyNums = input1 * input2;
var divideNums = input1 / input2;
var substractNums = input1 - input2;
document.write(`
<h1>Math with the numbers ${input1} and ${input2}</h1>
<p>${input1} + ${input2} = ${sumNums}</p>
<p>${input1} x ${input2} = ${multiplyNums}</p>
<p>${input1} / ${input2} = ${divideNums}</p>
<p>${input1} - ${input2} = ${substractNums}</p>
`);
First, I put the parseFloat in the same line of my variable input1, is that ok?
Second, I wrote all the output in the document.write
since I felt more comfortable this way, but would it be a good practice? I saw it in another video, just using ternary operators and breaking lines down to make it readable. But not sure if it's a good practice to do it within the document.write
function and not in a variable.
For example, assuming I'm getting an ID and I'm injecting HTML code like this, is that ok?
Thank you in advance :)
1 Answer
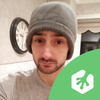
Jamie Reardon
Treehouse Project ReviewerYou are exactly like me when I first started learning JavaScript! First off, great job! You found and coded a working solution for the program! Second, I wouldn't worry so much right now about how you are coding individual statements like this. I did the same, and although it is great to care and think a lot about it, which in turn provides a better outcome of yourself as a developer, at this moment in time it really isn't needed. It is more important for you at this stage to get a strong grasp and understanding of the fundamental basics, knowing how the methods work etc! Also, in a later course, you will begin to find better ways to code small things like this through things like the D.R.Y. programming principles etc. So don't worry, just keep on coding, get the program you are trying to create to run the way you want it to, learn the concepts, then you can start to think twice about your code! :)