Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial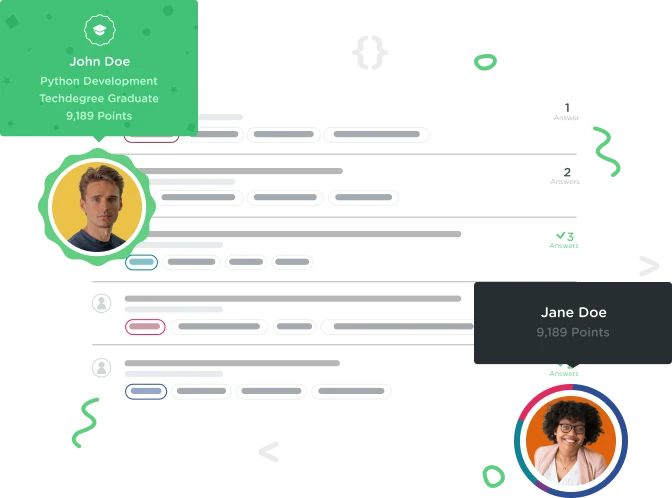
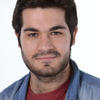
Sévak Kulinkian
1,086 PointsIs it a treehouse.com bug on this exercise?
Hey,
I don't know why my code is not working for treehouse.com work checker ... In my terminal when I execute my code I get exactly what the exercice ask for!
Maybe someone can guess why this is not working on the website ...
FYI, the exercise is the following: "You're on fire! Last one and it is, of course, the hardest. Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse. For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1]. You can do it!"
Thanks all for your help
def first_4(iterable):
return iterable[:4]
def last_4(iterable):
return iterable[-1:-5:-1]
def first_and_last_4(iterable):
the_first_4 = first_4(iterable)
the_last_4 = last_4(iterable)
return the_first_4 + list(reversed(the_last_4))
print(the_first_4 + reversed(the_last_4))
def odds(iterable):
new_iterable = []
odd_numbers = [2*x+1 for x in range(len(iterable)) if 2*x+1 < len(iterable)]
for n in odd_numbers:
item_indexed = iterable[n]
new_iterable.append(item_indexed)
return new_iterable
def reverse_evens(iterable):
new_iterable = []
even_numbers = [-(2*x+1) for x in range(len(iterable)) if (2*x+1) <= len(iterable)]
for n in even_numbers:
item_indexed = iterable[n]
new_iterable.append(item_indexed)
return new_iterable
x = odds([1,2,3,4,5,6,7,8,9,10,11,12,13,14,15])
y = first_and_last_4([1,2,3,4,5,6,7,8,9,10,11,12,13,14,15])
z = reverse_evens([1, 2, 3, 4, 5])
k = reverse_evens([1,2,3,4,5,6,7,8,9,10,11,12,13,14,15])
print(x)
print(y)
print(z)
print(k)
5 Answers
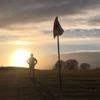
Stuart Wright
41,118 PointsThis is not a Treehouse bug. Notice that both of your test cases contain an odd number of elements. Try one with an even number of elements and you'll see that your function is not producing the correct output:
Passing [1, 2, 3, 4, 5, 6] to your function returns [6, 4, 2], and those numbers are all at an odd index.
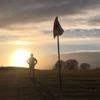
Stuart Wright
41,118 PointsChris - there are two issues with your code. One I assume is a simple typo, and the other relates to what the challenge is asking you to do.
The typo is that you missed the = sign between reverse and iterable here:
reverse iterable[::-1]
Edit to add: I see you noticed the above error yourself.
However, even after correcting that error, notice that you're first reversing the numbers, then filtering based on the new index, which might be different from the original index. You need to change the order of these two steps. Filter for even index elements first, then reverse:
def reverse_evens(iterable):
evens = iterable[::2]
return evens[::-1]
Chris Grazioli
31,225 PointsStuart, my code passes, which is why I can't see how this is an exercise worth doing. Its like the question wants you to find numbers in the even index, regardless of whether thay are actually even or not? so a list [0,1,1,2,3,4,5,6,7,8,9,10] would screw everything up
Chris Grazioli
31,225 PointsI'm having some trouble on the last one
def first_4(iterable):
return iterable[0:4]
def first_and_last_4(iterable):
first=iterable[0:4]
last=iterable[-4:]
return first+last
def odds(iterable):
return iterable[1::2]
def reverse_evens(iterable):
reverse iterable[::-1]
return reverse[0::2]
I'm not seeing what I'm doing wrong here
Chris Grazioli
31,225 Pointscorrections aside,
def first_4(iterable):
return iterable[0:4]
def first_and_last_4(iterable):
first=iterable[0:4]
last=iterable[-4:]
return first+last
def odds(iterable):
return iterable[1::2]
def reverse_evens(iterable):
reverse=iterable[::-1]
return reverse[0::2]
Chris Grazioli
31,225 PointsSOLVED
I have to say this one kinda sucked a bit, a little hint as to the number of items in the list affecting the way your function would work would have been helpful. Also... a practical application as to where you would ever use this would go along way
def first_4(iterable):
return iterable[0:4]
def first_and_last_4(iterable):
first=iterable[0:4]
last=iterable[-4:]
return first+last
def odds(iterable):
return iterable[1::2]
def reverse_evens(iterable):
#when the iterable contains an odd number of items
if len(iterable)%2 != 0:
return (iterable[::-1])[::2]
#when the iterable contains an even number of items
else:
return (iterable[::-1])[1::2]