Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial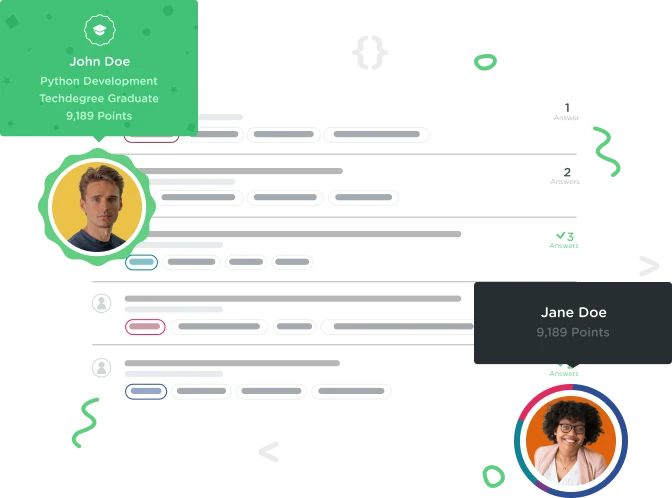
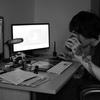
Andrew Robinson
16,372 PointsIs it better to create a variable for html string or use .createElement()?
Before watching the videos I attempted the task myself and wrote it using .createElement() and .addClass() etc, is it better to create a string like Dave did and then set the innerHTML, or are both just as efficient?
Here is the JavaScript I used:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if(xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var employeeList = document.getElementById('employeeList');
var employeeUl = document.createElement('ul');
employeeUl.classList.add('bulleted');
for (var i = 0; i < employees.length; i++) {
var employeeLi = document.createElement('li');
if(employees[i].inoffice) {
employeeLi.classList.add('in');
} else {
employeeLi.classList.add('out');
}
employeeLi.innerText = employees[i].name;
employeeUl.appendChild(employeeLi);
}
employeeList.appendChild(employeeUl);
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
Thanks,
Andy
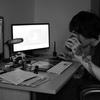
Andrew Robinson
16,372 PointsCheers for the link Dan, so if I understand correctly, createElement is better for Safari and Chrome (according to that graph it says higher is better) and innerHTML is slightly better for Firefox?
I did a CPU profile using dev tools and the createElement (in Chrome) was ~160ms faster for me, I think if I just wanted to create a paragraph or something static, i'd use innerHTML but in this case I like the createElement method.

Dan Oswalt
23,438 PointsMy takeaway was mainly that its a case where readable, manageable code trumps whatever minor optimization you might get.
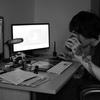
Andrew Robinson
16,372 PointsI certainly agree on the readability factor :)
3 Answers
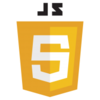
Ygor Fonseca
6,929 PointsHey Andrew, my solution was the same, I think the code becomes much more readable using 'createElement' than a string variable...
Here's how I did: https://w.trhou.se/kmvv5xo87e
Dan, thanks for the link...

sibal gesekki
3,484 Pointsheres mine using dom manipulation:
var x = new XMLHttpRequest();
x.onreadystatechange = function() {
if (x.readyState === 4 && x.status === 200) {
var parseResponse = JSON.parse(x.responseText);
var roomStatuses = coolFunc(parseResponse);
document.getElementById('roomList').appendChild(roomStatuses);
}
}
function coolFunc(rooms) {
var ul = document.createElement('ul');
ul.className = 'rooms'
for (var i=0, n=rooms.length; i<n; i++) {
var li = document.createElement('li');
var roomNum = document.createTextNode(rooms[i].room);
li.appendChild(roomNum);
if (rooms[i].available) {
li.className = 'empty';
} else {
li.className = 'full';
}
ul.appendChild(li);
ul.appendChild(document.createElement('br'));
}
return ul;
}
x.open('GET', '../data/rooms.json');
x.send();
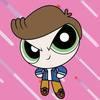
Cezary Burzykowski
18,291 PointsHey guys!
I did excactly the same thing as Andrew (to be honest my code was mirroring Adrews code). I think it's much more readable this way. Unfortunately I have encountered a problem. I had multiple errors for example when I was trying to append <ul>
to the <div>
it the same manner as Andrew did:
var parseResponse = JSON.parse(x.responseText);
var roomStatuses = coolFunc(parseResponse);
document.getElementById('roomList').appendChild(roomStatuses);
I got an error "document.getElementById('roomList') is not of type Node". I've checked the type of "document.getElementById('roomList')" and console showed me that it is an object and I'm pretty sure that
document.getElementById
shouldn't return an object ....
Dan Oswalt
23,438 PointsDan Oswalt
23,438 PointsInteresting. I don't know! But here's a good stackoverflow discussion about it:
http://stackoverflow.com/questions/15182402/javascript-document-createelement-or-html-tags