Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial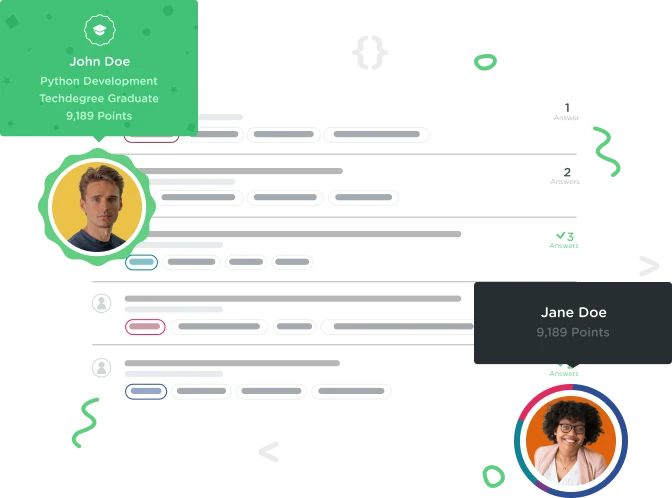
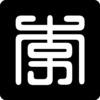
lihaoquan
12,045 PointsIs it better to update a specific property using setState(), or dump the whole state into setState()?
In the onScoreChange function, the player's score is changed by doing
this.state.players[index].score += delta;
this.setState(this.state);
But what if state contains some properties that do not need to be updated? Is it better to only let setState to change players.score property when calling setState() function instead of passing the whole this.state into the setState() function?
Will this affect performance if there are other properties in state as the application grows bigger?
4 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsI think a better way to change the state is to create a local variable containing the current property of the state you wish to change, and then update that and use it with setState
:
var players = this.state.players;
players[index].score += delta;
this.setState({players: players});
Or if you're using ES2015/ES6:
let {players} = this.state;
players[index].score += delta;
this.setState({players});

Laurie Williams
10,174 PointsThere's no reason that you can't dump the entire state but as the state gets bigger, the harder it will be to maintain.

Guil Hernandez
Treehouse TeacherAnother way you could update the score state is:
onScoreChange(index, delta) {
this.setState( prevState => {
return {
score: prevState.players[index].score += delta
};
});
}
use a second form of setState() that accepts a function rather than an object. That function will receive the previous state as the first argument, and the props at the time the update is applied as the second argument:

Sum Tsui
Full Stack JavaScript Techdegree Student 29,117 Pointsjust found out passing different arguments into setState()
and change score, then check State in devtool, the state will be different for:
this.setState(this.state);
this.setState(this.state.players);
this.setState(this.state.players[index]);
so maybe there is a reason to better passing the whole state.
hum4n01d
25,493 Pointshum4n01d
25,493 PointsCould you explain why this method is better Iain Simmons
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 Pointshum4n01d:
According to the React State and Lifecycle docs, the object you pass to
setState
is merged with the current state, so if you're passing the wholestate
object, it has to merge everything, and check all properties in the state to see what has changed.if you only pass the property you're changing, it only updates that property and leaves the rest as it is.
Also, changes to state happen asynchronously, so the state could be changing in the middle of the code where you're changing one property on the state object, and then calling
setState
. If you assign the current state or part of state that you're changing to another variable, you're essentially getting the value at that particular moment and ensuring that the changes you make to that value are the ones being merged with the rest of the state.Hope that makes sense.
hum4n01d
25,493 Pointshum4n01d
25,493 PointsOk, thanks Iain Simmons
M Ashraful Alam
12,328 PointsM Ashraful Alam
12,328 PointsGood point. Instead of creating a new variable we could just update state like -