Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial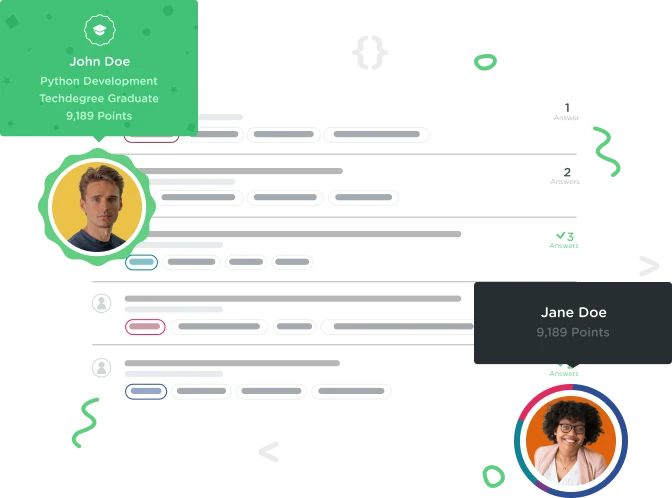
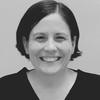
Angela Anders
1,844 PointsIs it considered good practice to call a function without defining it first?
For example in the program that we are doing here the show_list function is called before it is defined. I find it confusing, but if this is done frequently, I'll go with it.
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear_screen()
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should I add {} in?\n"
"Press ENTER to add to the end of the list\n"
"> ".format(item))
else:
position = 0
try:
postion = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear_screen()
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}, {}".format(index, item))
index += 1
print("-"* 10)
show_help()
while True:
new_item = input("> ")
if new_item.upper() == 'DONE' or new_item.upper == 'QUIT':
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'SHOW':
show_list()
continue
else:
add_to_list(new_item)
show_list()
2 Answers
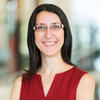
Louise St. Germain
19,424 PointsHi Angela,
I can see how it would be confusing!
However, one thing that should be noted, and which might clear things up a bit, is that when a function is defined (any chunk of code that starts with def <some_function_name>:
), Python is not actually executing that code while you are defining it. To keep the explanation very simple for now, when you define functions like this, it's storing the function in memory so it can use it later, but isn't actually running the code inside the function at that point.
So in your above example, when you run the file, it's going to:
- Import that
os
module - Create the empty list called shopping_list
- Take note of what you want done if someone calls clear_screen, show_help, add_to_list, or show_list (i.e., store all that info for later use)
Then it makes its first actual function call on the line:
show_help()
It runs the code inside show_help()
and goes through the while loop. add_to_list
doesn't get called until the last line of that while loop (if/when it gets there). By then, show_list
has already been defined, so everything runs smoothly.
There would be a problem if, say, you took that show_list
function and put it after the while loop. In that case, it would be true that add_to_list
would be pointing to a function that hasn't been defined yet, and Python will throw an error. (You can experiment with this in Workspaces if you like.)
I hope that helps a bit!
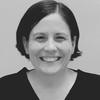
Angela Anders
1,844 PointsIt helps a lot! Things have to make logical sense for me to understand them and this does when you break it down Barney style. Thanks!